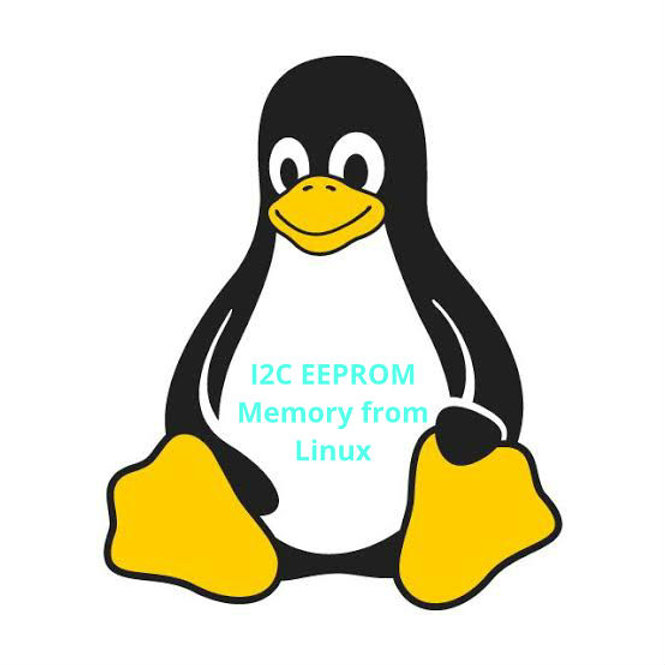
Accessing I2C EEPROM Memory from Linux
May 30, 2024
2 min read
3
244
I2C Electrically Erasable Programmable Read-Only Memory (EEPROM) devices play a crucial role in storing configuration data, calibration parameters, and other essential information in embedded systems. Linux provides robust support for accessing I2C EEPROM memory through the I2C subsystem, enabling developers to read, write, and manage data stored in EEPROM devices seamlessly. This article offers a detailed guide on accessing I2C EEPROM memory from Linux, covering device configuration, kernel driver selection, sysfs interface usage, and example code snippets.
1. Understanding I2C EEPROM Devices:
I2C EEPROM devices utilize the Inter-Integrated Circuit (I2C) protocol for communication, featuring byte-addressable memory cells that retain data even when powered off. These devices typically offer various capacities and interfaces, providing flexible storage options for embedded systems.
2. Device Configuration:
Before accessing an I2C EEPROM device from Linux, ensure proper device configuration, including setting the device address, bus speed, and other parameters. Device datasheets and documentation provide essential information for configuring I2C EEPROM devices according to the system requirements.
3. Kernel Driver Selection:
Linux offers several kernel drivers for managing I2C EEPROM devices, including generic drivers like "eeprom" and vendor-specific drivers tailored to specific EEPROM chipsets. Choose an appropriate kernel driver based on the target EEPROM device and system requirements.
4. Sysfs Interface Usage:
The sysfs interface in Linux provides a convenient mechanism for interacting with I2C EEPROM devices, offering virtual files for reading and writing data to EEPROM memory. By navigating the sysfs hierarchy, users can access and manipulate EEPROM data using standard file I/O operations.
5. Example Code Snippets:
Below are example code snippets demonstrating how to read and write data to an I2C EEPROM device from a Linux userspace application:
Example 1: Reading Data from I2C EEPROM
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define EEPROM_ADDRESS 0x50
#define BUFFER_SIZE 256
int main()
{
int file;
char buffer[BUFFER_SIZE];
// Open I2C bus
file = open("/dev/i2c-0", O_RDWR);
if (file < 0) {
perror("Failed to open I2C bus");
return 1;
}
// Set EEPROM address
if (ioctl(file, I2C_SLAVE, EEPROM_ADDRESS) < 0) {
perror("Failed to set I2C address");
close(file);
return 1;
}
// Read data from EEPROM
if (read(file, buffer, BUFFER_SIZE) != BUFFER_SIZE) {
perror("Failed to read from EEPROM");
close(file);
return 1;
}
// Display read data
printf("Data read from EEPROM: %s\n", buffer);
close(file);
return 0;
}
Example 2: Writing Data to I2C EEPROM
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define EEPROM_ADDRESS 0x50
#define DATA_TO_WRITE "Hello, I2C EEPROM!"
int main()
{
int file;
// Open I2C bus
file = open("/dev/i2c-0", O_RDWR);
if (file < 0) {
perror("Failed to open I2C bus");
return 1;
}
// Set EEPROM address
if (ioctl(file, I2C_SLAVE, EEPROM_ADDRESS) < 0) {
perror("Failed to set I2C address");
close(file);
return 1;
}
// Write data to EEPROM
if (write(file, DATA_TO_WRITE, sizeof(DATA_TO_WRITE)) != sizeof(DATA_TO_WRITE)) {
perror("Failed to write to EEPROM");
close(file);
return 1;
}
printf("Data written to EEPROM successfully\n");
close(file);
return 0;
}
Accessing I2C EEPROM memory from Linux involves configuring the device, selecting the appropriate kernel driver, and leveraging the sysfs interface for interacting with EEPROM data. With the provided example code snippets and guidance, developers can seamlessly integrate I2C EEPROM devices into Linux-based embedded systems, enabling reliable and efficient data storage solutions.
#linuxdevicedrivers #ldd #linuxlovers
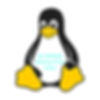