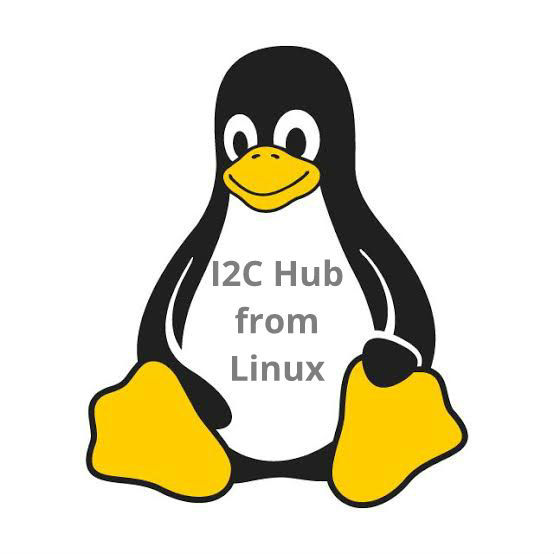
Accessing I2C Hub from Linux
- mkmints
- Jun 1, 2024
- 3 min read
I2C hubs are essential components in embedded systems, enabling the connection of multiple I2C devices to a single I2C bus. These hubs act as signal amplifiers, extenders, or multiplexers, facilitating efficient communication between the host controller and various I2C peripherals. This article serves as a comprehensive guide on accessing I2C hubs from Linux, covering device detection, driver selection, sysfs interface usage, and example code snippets.
1. Understanding I2C Hubs:
I2C hubs, also known as I2C splitters or multiplexers, are devices designed to expand the I2C bus's capabilities by allowing multiple devices to communicate over a single bus. These hubs typically feature multiple input/output ports, each connected to a separate I2C device. They help overcome limitations such as bus capacitance and signal degradation, ensuring reliable communication between the host and peripheral devices.
2. Device Detection and Identification:
Before accessing an I2C hub from Linux, it's crucial to detect and identify the hub connected to the I2C bus. Linux provides utilities like "i2cdetect" to scan the I2C bus and identify active devices. By running "i2cdetect -y <bus_number>", users can identify the address of the connected I2C hub.
3. Kernel Driver Selection:
Linux offers various kernel drivers for managing I2C hubs, including generic drivers like "i2c-mux" and vendor-specific drivers tailored to specific hub ICs. Choose an appropriate kernel driver based on the target I2C hub and system requirements.
4. Sysfs Interface Usage:
The sysfs interface in Linux provides a convenient mechanism for interacting with I2C hubs, offering virtual files for configuring hub channels, selecting active channels, and monitoring hub status. By navigating the sysfs hierarchy, users can access and manipulate hub channels using standard file I/O operations.
5. Example Code Snippets:
Below are example code snippets demonstrating how to configure and control an I2C hub from a Linux userspace application:
Example 1: Configuring Hub Channels on an I2C Hub
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define I2C_HUB_ADDRESS 0x71
int main()
{
int file;
unsigned char channel_config = 0x0F; // Enable all four channels
// Open I2C bus
file = open("/dev/i2c-0", O_RDWR);
if (file < 0) {
perror("Failed to open I2C bus");
return 1;
}
// Set I2C hub address
if (ioctl(file, I2C_SLAVE, I2C_HUB_ADDRESS) < 0) {
perror("Failed to set I2C address");
close(file);
return 1;
}
// Write channel configuration to the hub
if (write(file, &channel_config, sizeof(channel_config)) != sizeof(channel_config)) {
perror("Failed to configure hub channels");
close(file);
return 1;
}
printf("I2C Hub Channels Configured Successfully\n");
close(file);
return 0;
}
Example 2: Selecting Active Channel on an I2C Hub
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define I2C_HUB_ADDRESS 0x71
int main()
{
int file;
unsigned char active_channel = 0x01; // Select channel 1
// Open I2C bus
file = open("/dev/i2c-0", O_RDWR);
if (file < 0) {
perror("Failed to open I2C bus");
return 1;
}
// Set I2C hub address
if (ioctl(file, I2C_SLAVE, I2C_HUB_ADDRESS) < 0) {
perror("Failed to set I2C address");
close(file);
return 1;
}
// Write active channel selection to the hub
if (write(file, &active_channel, sizeof(active_channel)) != sizeof(active_channel)) {
perror("Failed to select active channel");
close(file);
return 1;
}
printf("Active Channel Selected Successfully\n");
close(file);
return 0;
}
Accessing I2C hubs from Linux involves device detection, kernel driver selection, and leveraging the sysfs interface for channel configuration and channel selection. With the provided guidance and example code snippets, developers can effectively integrate I2C hubs into Linux-based embedded systems, enabling efficient communication with multiple I2C peripherals over a single bus.
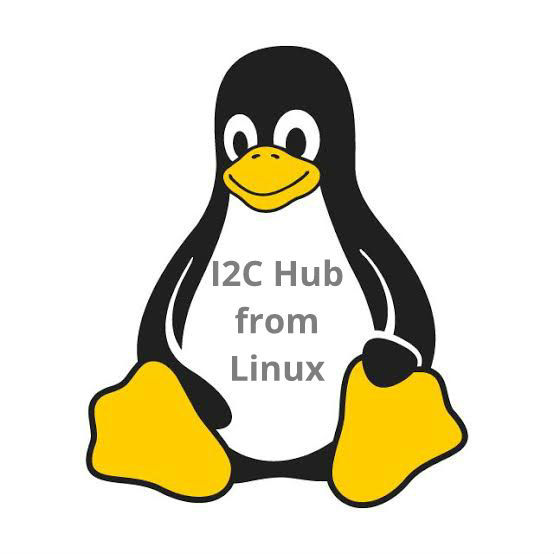