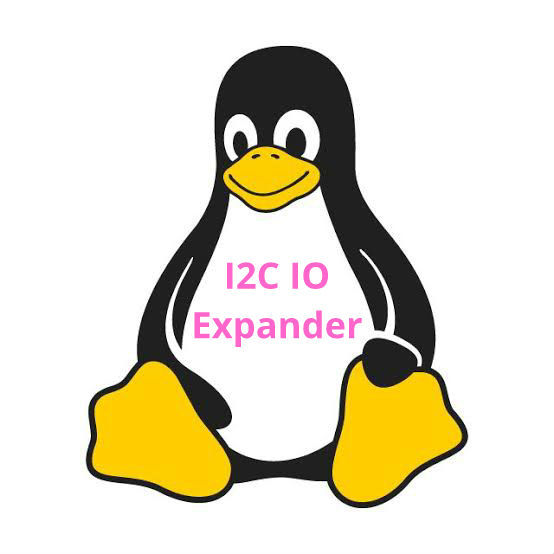
Accessing I2C IO Expander from Linux
- mkmints
- Jun 1, 2024
- 3 min read
I2C IO expanders are versatile devices commonly used in embedded systems to increase the number of GPIO (General Purpose Input/Output) pins available for interfacing with external components. These expanders provide a convenient way to control multiple digital inputs and outputs using the Inter-Integrated Circuit (I2C) communication protocol. This article serves as a comprehensive guide on accessing I2C IO expanders from Linux, covering device detection, driver selection, sysfs interface usage, and example code snippets.
1. Understanding I2C IO Expanders:
I2C IO expanders are ICs designed to extend the number of GPIO pins available to a microcontroller or embedded system. They typically feature multiple input/output ports that can be configured as either inputs or outputs, allowing for flexible digital interfacing with external sensors, actuators, and other peripherals. These expanders communicate with the host controller via the I2C bus, enabling seamless integration into Linux-based systems.
2. Device Detection and Identification:
Before accessing an I2C IO expander from Linux, it's essential to detect and identify the expander connected to the I2C bus. Linux provides utilities like "i2cdetect" to scan the I2C bus and identify active devices. By running "i2cdetect -y <bus_number>", users can identify the address of the connected I2C IO expander.
3. Kernel Driver Selection:
Linux offers several kernel drivers for managing I2C IO expanders, including generic drivers like "gpio-pca953x" and vendor-specific drivers tailored to specific IO expander ICs. Choose an appropriate kernel driver based on the target IO expander and system requirements.
4. Sysfs Interface Usage:
The sysfs interface in Linux provides a convenient mechanism for interacting with I2C IO expanders, offering virtual files for configuring GPIO direction, setting output values, and monitoring input states. By navigating the sysfs hierarchy, users can access and manipulate GPIO pins using standard file I/O operations.
5. Example Code Snippets:
Below are example code snippets demonstrating how to configure GPIO pins and read/write digital values using an I2C IO expander from a Linux userspace application:
Example 1: Configuring GPIO Direction (Output/Input) on an I2C IO Expander
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define IO_EXPANDER_ADDRESS 0x20
int main()
{
int file;
unsigned char config_byte = 0x00; // Set all GPIO pins as outputs
// Open I2C bus
file = open("/dev/i2c-0", O_RDWR);
if (file < 0) {
perror("Failed to open I2C bus");
return 1;
}
// Set IO expander address
if (ioctl(file, I2C_SLAVE, IO_EXPANDER_ADDRESS) < 0) {
perror("Failed to set I2C address");
close(file);
return 1;
}
// Write configuration byte to set GPIO direction
if (write(file, &config_byte, sizeof(config_byte)) != sizeof(config_byte)) {
perror("Failed to configure GPIO direction");
close(file);
return 1;
}
printf("GPIO Direction Configured Successfully\n");
close(file);
return 0;
}
Example 2: Reading Input States from an I2C IO Expander
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define IO_EXPANDER_ADDRESS 0x20
int main()
{
int file;
unsigned char input_state;
// Open I2C bus
file = open("/dev/i2c-0", O_RDWR);
if (file < 0) {
perror("Failed to open I2C bus");
return 1;
}
// Set IO expander address
if (ioctl(file, I2C_SLAVE, IO_EXPANDER_ADDRESS) < 0) {
perror("Failed to set I2C address");
close(file);
return 1;
}
// Read input state from GPIO pins
if (read(file, &input_state, sizeof(input_state)) != sizeof(input_state)) {
perror("Failed to read GPIO input state");
close(file);
return 1;
}
printf("GPIO Input State: %02x\n", input_state);
close(file);
return 0;
}
Accessing I2C IO expanders from Linux involves device detection, kernel driver selection, and leveraging the sysfs interface for GPIO configuration and manipulation. With the provided guidance and example code snippets, developers can effectively integrate I2C IO expanders into Linux-based embedded systems, enabling flexible digital interfacing with external components and peripherals.
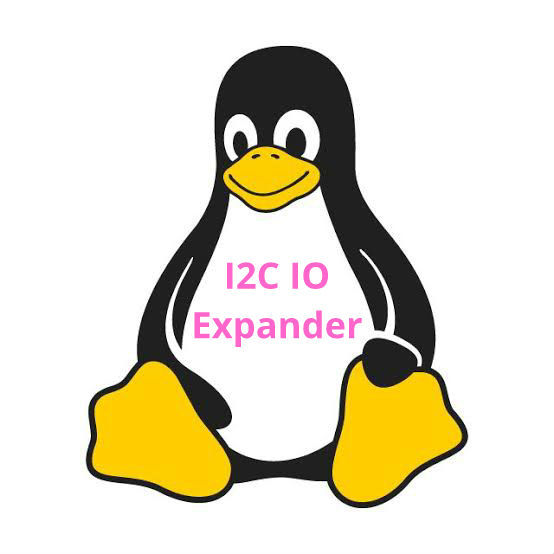