Accessing I2C Temperature Sensor from Linux
May 30, 2024
2 min read
1
158
I2C temperature sensors are essential components in embedded systems, providing accurate temperature measurement capabilities for various applications such as environmental monitoring, industrial automation, and consumer electronics. Linux offers robust support for interfacing with I2C temperature sensors, allowing developers to read temperature data, configure sensor settings, and integrate temperature sensing functionality into their applications seamlessly. This article serves as a comprehensive guide on accessing I2C temperature sensors from Linux, covering device detection, driver selection, sysfs interface usage, and example code snippets.
1. Understanding I2C Temperature Sensors:
I2C temperature sensors are semiconductor devices that measure temperature and provide digital temperature data via the Inter-Integrated Circuit (I2C) communication protocol. These sensors come in various forms, including standalone sensor modules and integrated sensor ICs, offering high accuracy, low power consumption, and small form factors suitable for diverse temperature sensing applications.
2. Device Detection and Identification:
Before accessing an I2C temperature sensor from Linux, it's essential to detect and identify the sensor connected to the I2C bus. Linux provides utilities like "i2cdetect" to scan the I2C bus and identify active devices. By running "i2cdetect -y <bus_number>", users can identify the address of the connected I2C temperature sensor.
3. Kernel Driver Selection:
Linux offers several kernel drivers for managing I2C temperature sensors, including generic drivers like "lm75" and vendor-specific drivers tailored to specific sensor ICs. Choose an appropriate kernel driver based on the target temperature sensor and system requirements.
4. Sysfs Interface Usage:
The sysfs interface in Linux provides a convenient mechanism for interacting with I2C temperature sensors, offering virtual files for reading temperature data, configuring sensor settings, and monitoring sensor status. By navigating the sysfs hierarchy, users can access and manipulate temperature sensor data using standard file I/O operations.
5. Example Code Snippets:
Below are example code snippets demonstrating how to read temperature data from an I2C temperature sensor using a Linux userspace application:
Example 1: Reading Temperature Data from I2C Sensor
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define SENSOR_ADDRESS 0x48
int main()
{
int file;
unsigned char buffer[2];
// Open I2C bus
file = open("/dev/i2c-0", O_RDWR);
if (file < 0) {
perror("Failed to open I2C bus");
return 1;
}
// Set sensor address
if (ioctl(file, I2C_SLAVE, SENSOR_ADDRESS) < 0) {
perror("Failed to set I2C address");
close(file);
return 1;
}
// Read temperature data
if (read(file, buffer, sizeof(buffer)) != sizeof(buffer)) {
perror("Failed to read temperature data");
close(file);
return 1;
}
// Convert raw data to temperature
int temperature = ((buffer[0] << 8) | buffer[1]) >> 4;
temperature *= 0.0625; // Conversion factor for LM75
printf("Temperature: %d°C\n", temperature);
close(file);
return 0;
}
Accessing I2C temperature sensors from Linux involves detecting the sensor, selecting the appropriate kernel driver, and leveraging the sysfs interface for interacting with sensor data. With the provided guidance and example code snippets, developers can integrate temperature sensing functionality into Linux-based embedded systems effectively, enabling accurate temperature monitoring and control in diverse applications.
#linuxdevicedrivers #ldd #linuxlovers
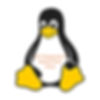