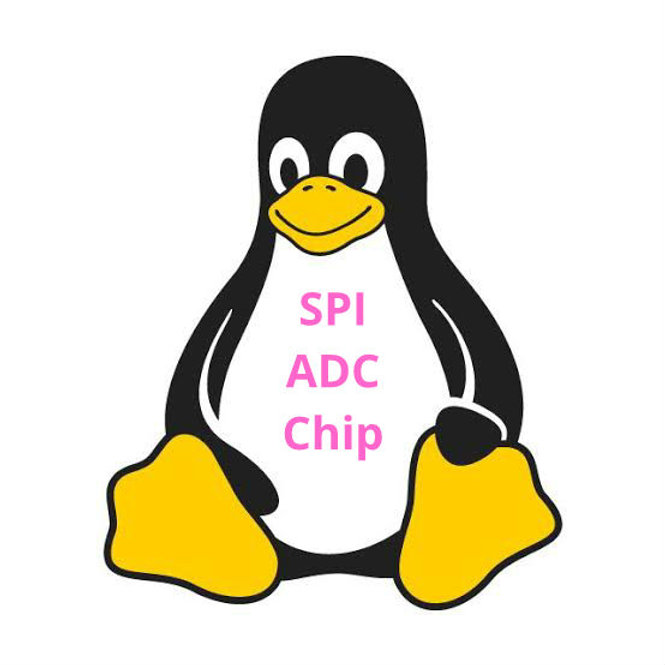
Serial Peripheral Interface (SPI) is a synchronous serial communication protocol commonly used for interfacing with analog-to-digital converters (ADCs), sensors, and other peripheral devices. This article serves as a comprehensive guide on accessing an SPI ADC chip from Linux, covering device configuration, kernel driver selection, SPI interface usage, and example code snippets.
1. Understanding SPI ADC Communication:
SPI ADC chips convert analog signals into digital data and communicate with the host controller (such as a microcontroller or a Linux-based system) over the SPI bus. Each ADC chip has configuration registers and data registers accessible via SPI transactions. SPI communication involves sending commands or address bytes followed by data bytes to read or write registers.
2. Device Configuration and Kernel Driver Selection:
Before accessing an SPI ADC chip from Linux, ensure that the SPI interface is enabled in the kernel configuration and the appropriate kernel drivers are available. Linux provides SPI kernel drivers for various SPI controllers and devices. Choose the suitable SPI driver for the SPI controller used in your system and configure it accordingly.
3. SPI Interface Usage:
The Linux SPI framework offers a user-friendly interface for interacting with SPI devices. The SPI device nodes are typically located under "/dev/spidev" in the filesystem. To communicate with the SPI ADC chip, open the corresponding SPI device node and perform SPI transactions using read and write operations.
4. Example Code Snippets:
Below are example code snippets demonstrating how to read analog voltage data from an SPI ADC chip (e.g., MCP3008) using Linux SPI interface:
Example 1: SPI Initialization and Configuration
// Open SPI device file
int spi_fd = open("/dev/spidevX.Y", O_RDWR);
if (spi_fd < 0) {
perror("Error opening SPI device");
exit(EXIT_FAILURE);
}
// Set SPI mode and clock frequency
uint8_t mode = SPI_MODE_0;
ioctl(spi_fd, SPI_IOC_WR_MODE, &mode);
// Set SPI word size (bits per word)
uint8_t bits_per_word = 8;
ioctl(spi_fd, SPI_IOC_WR_BITS_PER_WORD, &bits_per_word);
Example 2: Reading Analog Voltage Data from SPI ADC Chip (MCP3008)
// SPI transaction structure
struct spi_ioc_transfer spi_transfer = {
.tx_buf = (unsigned long)&tx_data,
.rx_buf = (unsigned long)&rx_data,
.len = sizeof(tx_data),
.speed_hz = 1000000, // SPI clock frequency (1 MHz)
};
// Send SPI transaction
if (ioctl(spi_fd, SPI_IOC_MESSAGE(1), &spi_transfer) < 0) {
perror("Error performing SPI transaction");
exit(EXIT_FAILURE);
}
// Process received data (analog voltage)
uint16_t analog_value = ((rx_data[1] & 0x03) << 8) | rx_data[2];
double voltage = (analog_value * 3.3) / 1023; // Assuming 3.3V reference voltage
Accessing SPI ADC chips from Linux involves device configuration, kernel driver selection, and utilizing the SPI interface for communication. By following the guidelines and example code snippets provided in this article, developers can effectively integrate SPI ADC chips into Linux-based systems, enabling analog-to-digital conversion and data acquisition with ease.
#linuxdevicedrivers #ldd #linuxlovers

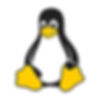