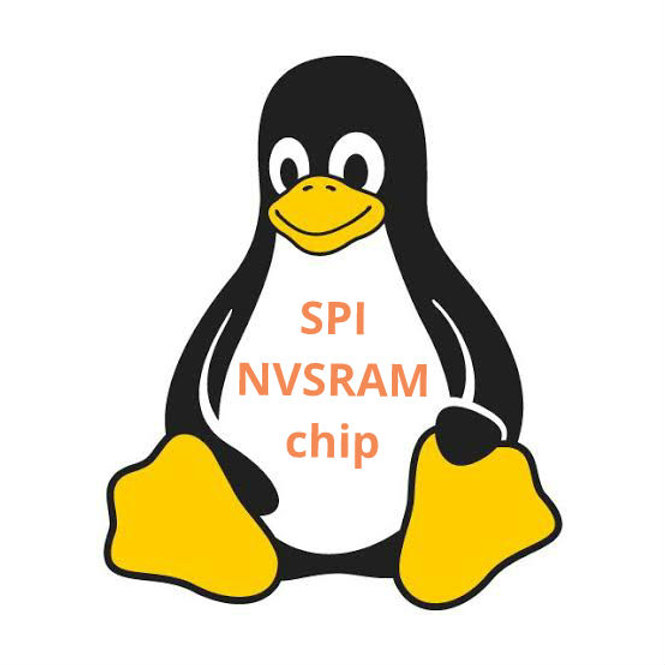
Serial Peripheral Interface (SPI) Non-Volatile Static Random Access Memory (NVSRAM) chips provide fast access to non-volatile memory storage via SPI communication. This article serves as a comprehensive guide on accessing an SPI NVSRAM chip from Linux, covering device detection, kernel driver selection, SPI interface usage, and example code snippets.
1. Understanding SPI NVSRAM Communication:
SPI NVSRAM chips combine the benefits of SRAM and non-volatile memory, offering fast read/write access and data retention during power loss. These chips typically have SPI-compatible interfaces, allowing direct communication with microcontrollers or embedded systems. SPI NVSRAM communication involves sending read/write commands and data bytes over the SPI bus.
2. Device Detection and Identification:
Before accessing an SPI NVSRAM chip from Linux, it's crucial to detect and identify the NVSRAM device connected to the SPI bus. Linux provides support for SPI devices through kernel drivers, which are responsible for detecting and configuring SPI devices. Users can use tools like "ls /sys/bus/spi/devices" to list detected SPI devices and their corresponding device nodes.
3. Kernel Driver Selection:
The appropriate kernel driver for the SPI NVSRAM chip must be selected and configured in the Linux kernel. Depending on the NVSRAM chip model and manufacturer, Linux may provide built-in support for specific NVSRAM drivers or generic SPI device drivers compatible with various NVSRAM chips. Ensure that the selected kernel driver supports the SPI NVSRAM chip used in the system.
4. SPI Interface Usage:
Once the SPI NVSRAM chip is detected, users can access it through the SPI interface using the SPI framework provided by the Linux kernel. SPI NVSRAM devices are typically accessed as SPI slave devices, with each NVSRAM chip having its unique chip select (CS) line. Users can open the corresponding SPI device node and perform SPI transactions to read from or write to the NVSRAM memory.
5. Example Code Snippets:
Below are example code snippets demonstrating how to read from and write to an SPI NVSRAM chip (e.g., Microchip 23LCV1024) using Linux SPI interface:
Example 1: Reading Data from SPI NVSRAM
// Open SPI device file
int spi_fd = open("/dev/spidevX.Y", O_RDWR);
if (spi_fd < 0) {
perror("Error opening SPI device");
exit(EXIT_FAILURE);
}
// Set SPI mode and clock frequency
uint8_t mode = SPI_MODE_0;
ioctl(spi_fd, SPI_IOC_WR_MODE, &mode);
// Set SPI word size (bits per word)
uint8_t bits_per_word = 8;
ioctl(spi_fd, SPI_IOC_WR_BITS_PER_WORD, &bits_per_word);
// Perform SPI read transaction to NVSRAM
uint8_t read_cmd = 0x03; // Read command byte
uint8_t read_data[PAGE_SIZE]; // Buffer for read data
struct spi_ioc_transfer spi_transfer = {
.tx_buf = (unsigned long)&read_cmd,
.rx_buf = (unsigned long)read_data,
.len = PAGE_SIZE, // Read page size
.speed_hz = 1000000, // SPI clock frequency (1 MHz)
};
if (ioctl(spi_fd, SPI_IOC_MESSAGE(1), &spi_transfer) < 0) {
perror("Error performing SPI read transaction");
exit(EXIT_FAILURE);
}
// Process read_data buffer
Example 2: Writing Data to SPI NVSRAM
// Open SPI device file
int spi_fd = open("/dev/spidevX.Y", O_RDWR);
if (spi_fd < 0) {
perror("Error opening SPI device");
exit(EXIT_FAILURE);
}
// Set SPI mode and clock frequency
uint8_t mode = SPI_MODE_0;
ioctl(spi_fd, SPI_IOC_WR_MODE, &mode);
// Set SPI word size (bits per word)
uint8_t bits_per_word = 8;
ioctl(spi_fd, SPI_IOC_WR_BITS_PER_WORD, &bits_per_word);
// Prepare write command and data
uint8_t write_cmd[3] = {0x02, 0x00, 0x00}; // Write command byte and address
uint8_t write_data[PAGE_SIZE]; // Data to be written
// Populate write_data buffer
// Perform SPI write transaction to NVSRAM
struct spi_ioc_transfer spi_transfer = {
.tx_buf = (unsigned long)write_cmd,
.rx_buf = NULL,
.len = sizeof(write_cmd),
.speed_hz = 1000000, // SPI clock frequency (1 MHz)
};
if (ioctl(spi_fd, SPI_IOC_MESSAGE(1), &spi_transfer) < 0) {
perror("Error performing SPI write command transaction");
exit(EXIT_FAILURE);
}
spi_transfer.tx_buf = (unsigned long)write_data;
spi_transfer.len = sizeof(write_data);
if (ioctl(spi_fd, SPI_IOC_MESSAGE(1), &spi_transfer) < 0) {
perror("Error performing SPI write data transaction");
exit(EXIT_FAILURE);
}
Accessing SPI NVSRAM chips from Linux involves device detection, kernel driver selection, and utilizing the SPI interface for communication. By following the guidelines and example code snippets provided in this article, developers can effectively read from and write to SPI NVSRAM chips in Linux-based systems, enabling data storage and retrieval applications in various embedded systems and IoT devices.
#linuxdevicedrivers #ldd #linuxlovers
