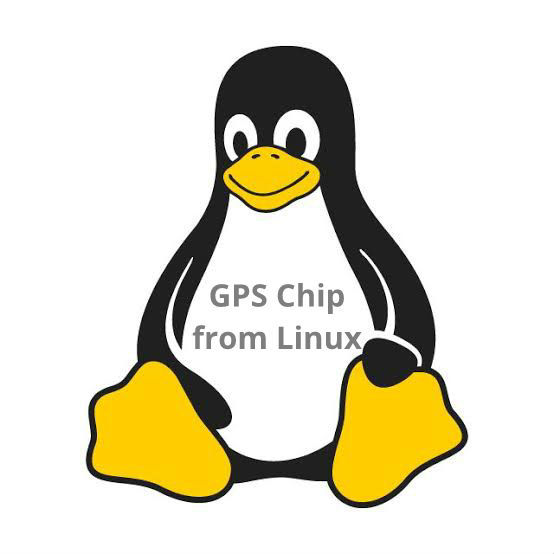
Accessing UART GPS Chip from Linux
- mkmints
- Jun 11, 2024
- 2 min read
Global Positioning System (GPS) chips with UART interfaces are commonly used in embedded systems for accurate location tracking. This article provides a detailed guide on accessing a UART GPS chip from Linux, covering device detection, UART configuration, data parsing, and example code snippets for communication.
1. Understanding UART GPS Communication:
UART GPS chips communicate using the Universal Asynchronous Receiver-Transmitter (UART) protocol, allowing serial data transmission between the GPS chip and the host system. GPS modules typically output NMEA (National Marine Electronics Association) sentences containing essential location and time information.
2. Device Detection and Identification:
Before accessing the UART GPS chip from Linux, it's essential to detect and identify the GPS device connected to the UART interface. Linux provides support for UART devices through serial drivers responsible for detecting and configuring UART devices. Tools like "dmesg" or "ls /dev/tty*" can help identify connected UART devices.
3. UART Configuration:
Once the UART GPS chip is detected, configure the UART interface settings such as baud rate, data bits, stop bits, and parity. The stty command-line tool or IOCTL calls in C/C++ can be used to set UART parameters. Ensure that the UART settings match the specifications of the GPS module to establish proper communication.
4. Data Parsing:
After configuring the UART interface, read data from the UART GPS chip to retrieve NMEA sentences containing location, time, and other relevant information. Parse the NMEA sentences to extract the required data fields using string manipulation or dedicated NMEA parsing libraries. Common NMEA sentences include GGA (Global Positioning System Fix Data), RMC (Recommended Minimum Specific GNSS Data), and GSA (GNSS DOP and Active Satellites).
5. Example Code Snippets:
Below are example code snippets demonstrating how to configure the UART interface and parse NMEA sentences from a UART GPS chip in Linux:
Example 1: UART Configuration in C/C++
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int main()
{
int uart_fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (uart_fd < 0) {
perror("Error opening UART device");
return -1;
}
struct termios options;
tcgetattr(uart_fd, &options);
// Set baud rate to 9600
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
// Set data bits to 8, no parity, 1 stop bit
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(uart_fd, TCSANOW, &options);
close(uart_fd);
return 0;
}
Example 2: Reading NMEA Sentences in C/C++
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#define BUFFER_SIZE 256
int main()
{
int uart_fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (uart_fd < 0) {
perror("Error opening UART device");
return -1;
}
char buffer[BUFFER_SIZE];
ssize_t bytes_read;
// Read data from UART
while ((bytes_read = read(uart_fd, buffer, BUFFER_SIZE)) > 0) {
// Process NMEA sentences in buffer
// Example: printf("Received data: %s\n", buffer);
}
close(uart_fd);
return 0;
}
Accessing UART GPS chips from Linux involves device detection, UART configuration, and data parsing of NMEA sentences. By following the guidelines and example code snippets provided in this article, developers can effectively communicate with UART GPS modules in Linux-based systems, enabling accurate location tracking and navigation functionalities.
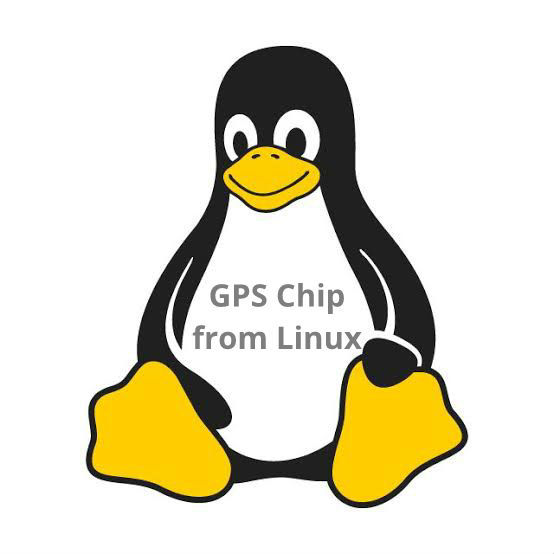