
Advanced Pthread APIs in Linux
- mkmints
- May 5, 2024
- 2 min read
Pthreads, or POSIX threads, offer a wealth of functionality beyond basic thread creation and synchronization. In this article, we'll explore some of the more advanced pthread APIs provided by the Linux kernel, including thread attributes, thread-specific data, and thread cancellation, along with detailed examples to illustrate their usage.
1. Thread Attributes:
The pthread library provides functions for manipulating thread attributes, allowing developers to customize various aspects of thread behavior such as scheduling policy, stack size, and priority. Here's an example demonstrating the use of thread attributes to set a custom stack size:
#include <pthread.h>
void* thread_function(void* arg) {
// Thread logic
return NULL;
}
int main() {
pthread_attr_t attr;
pthread_attr_init(&attr);
size_t stack_size = 1024 * 1024; // 1 MB
pthread_attr_setstacksize(&attr, stack_size);
pthread_t thread;
pthread_create(&thread, &attr, thread_function, NULL);
pthread_attr_destroy(&attr);
pthread_exit(NULL);
}
2. Thread-Specific Data:
Pthreads support thread-specific data, allowing each thread to have its own unique data associated with it. This can be useful for scenarios where global data is not appropriate. Here's an example demonstrating the use of thread-specific data:
#include <pthread.h>
pthread_key_t key;
pthread_once_t key_once = PTHREAD_ONCE_INIT;
void destructor(void* value) {
// Cleanup function
free(value);
}
void create_key() {
pthread_key_create(&key, destructor);
}
void* thread_function(void* arg) {
pthread_once(&key_once, create_key);
int* data = (int*)malloc(sizeof(int));
*data = 42;
pthread_setspecific(key, data);
return NULL;
}
int main() {
pthread_t thread;
pthread_create(&thread, NULL, thread_function, NULL);
pthread_join(thread, NULL);
int* data = pthread_getspecific(key);
printf("Thread-specific data: %d\n", *data);
pthread_key_delete(key);
return 0;
}
3. Thread Cancellation:
Pthreads support thread cancellation, allowing one thread to terminate another thread's execution. This feature can be used to implement cooperative thread termination or to handle exceptional conditions. Here's an example demonstrating thread cancellation:
#include <pthread.h>
void* thread_function(void* arg) {
while (1) {
// Thread logic
pthread_testcancel(); // Check for cancellation
}
pthread_exit(NULL);
}
int main() {
pthread_t thread;
pthread_create(&thread, NULL, thread_function, NULL);
// Cancel thread after 5 seconds
sleep(5);
pthread_cancel(thread);
pthread_join(thread, NULL);
return 0;
}
In this article, we've explored some of the advanced pthread APIs provided by the Linux kernel, including thread attributes, thread-specific data, and thread cancellation. By mastering these APIs, developers can unlock the full potential of multithreading in Linux, building robust and scalable applications. Experiment with the provided examples to deepen your understanding and proficiency in pthread programming.
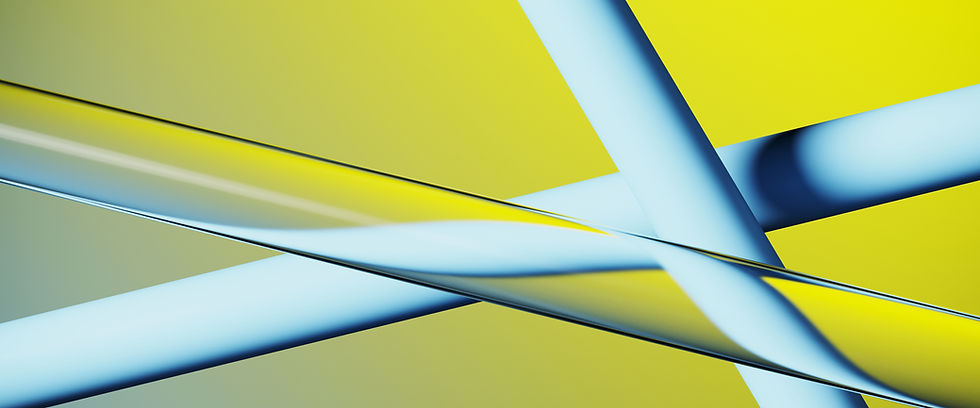