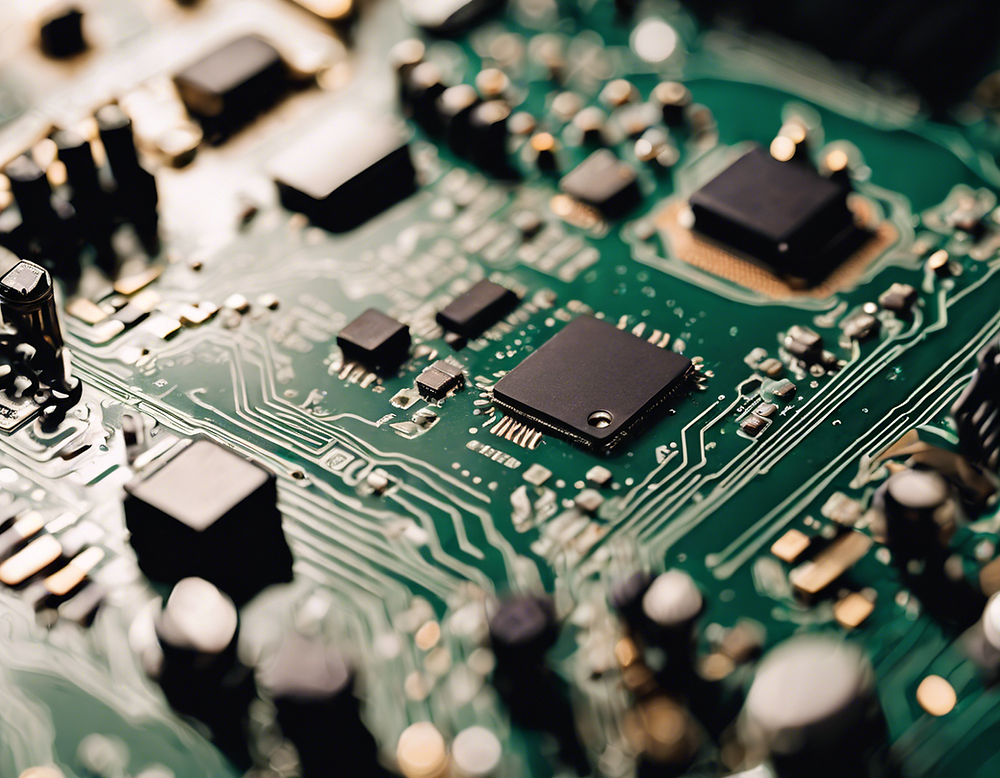
1. PCI Device Enumeration:
- When the Linux kernel boots up, it enumerates all PCI devices present in the system. We can iterate through each PCI device using the `pci_for_each_dev` macro provided by the kernel. Here's an example code snippet that demonstrates how to enumerate PCI devices and print their vendor and device IDs:
#include <linux/pci.h>
void enumerate_pci_devices(void)
{
struct pci_dev *dev;
/* Iterate through each PCI device */
pci_for_each_dev(dev) {
/* Read device vendor and device IDs */
u16 vendor_id = pci_read_config_word(dev, PCI_VENDOR_ID);
u16 device_id = pci_read_config_word(dev, PCI_DEVICE_ID);
/* Print device information */
pr_info("PCI Device: Vendor ID = 0x%x, Device ID = 0x%x\n", vendor_id, device_id);
}
}
2. Device Configuration Space:
- Each PCI device has a configuration space consisting of configuration registers that hold vital information about the device. We can read and write these registers using the `pci_read_config_*` and `pci_write_config_*` functions provided by the kernel. Here's an example code snippet that accesses the PCI command register of a device:
#include <linux/pci.h>
void access_device_config_space(struct pci_dev *pdev)
{
u32 value;
/* Read device configuration register */
value = pci_read_config_dword(pdev, PCI_COMMAND);
pr_info("PCI Command register value: 0x%x\n", value);
/* Write to device configuration register */
pci_write_config_dword(pdev, PCI_COMMAND, value | PCI_COMMAND_MEMORY);
}
3. Device Initialization Sequence:
- Initializing a PCI device involves resetting the device, configuring its registers, and enabling it for operation. Here's an example code snippet that illustrates the device initialization process:
#include <linux/pci.h>
void initialize_pci_device(struct pci_dev *pdev)
{
/* Reset the device */
pci_reset_device(pdev);
/* Configure base address registers (BARs) */
pci_write_config_dword(pdev, PCI_BASE_ADDRESS_0, 0x12345678);
/* Enable the device */
pci_enable_device(pdev);
}
#linuxdevicedrivers #ldd #linuxlovers
