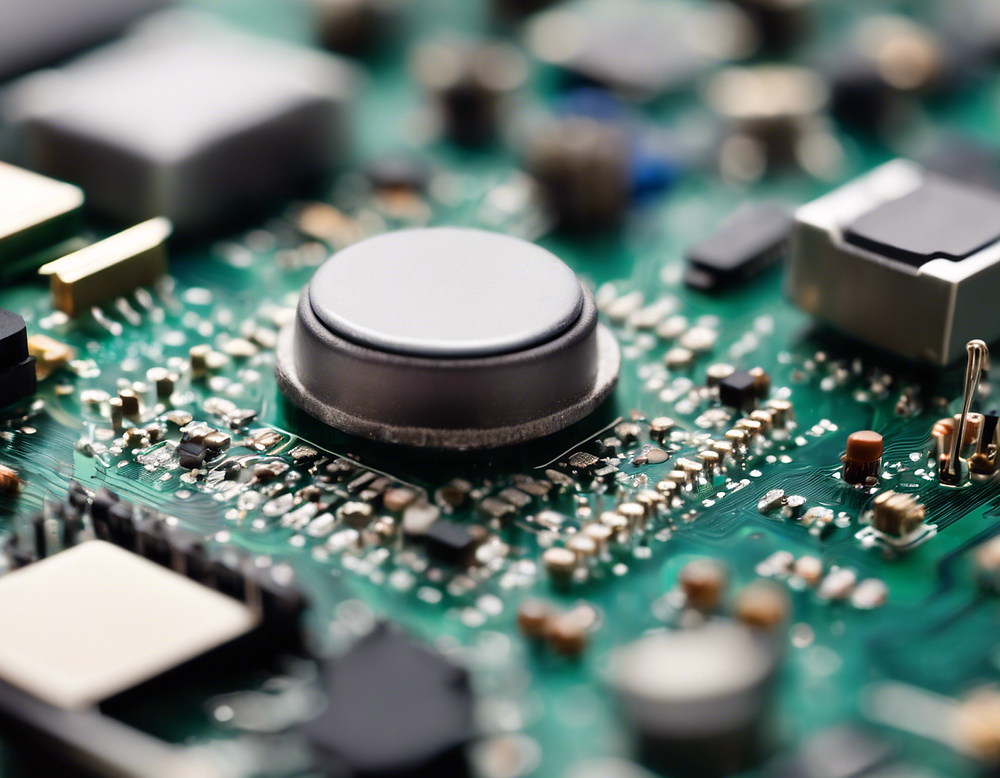
In this next part, we will delve deeper into advanced topics related to PCI device management and driver development in Linux.
1. Error Handling and Recovery:
- Error handling is crucial for robust device operation. In the event of errors, devices need to be able to recover gracefully to prevent system instability. Here's an example code snippet that demonstrates error handling and recovery techniques for PCI devices:
#include <linux/pci.h>
void handle_pci_errors(struct pci_dev *pdev)
{
/* Check for device errors */
if (pci_channel_offline(pdev)) {
pr_err("PCI channel offline, resetting device...\n");
pci_reset_device(pdev);
}
}
2. Hotplug Support:
- Hotplug support allows PCI devices to be dynamically added or removed from the system while it is running. This feature is especially useful in server environments where hardware components may need to be replaced without shutting down the system. Here's an example code snippet that demonstrates hotplug event handling for PCI devices:
#include <linux/pci.h>
void handle_pci_hotplug(struct pci_dev *pdev)
{
/* Check if device is being hotplugged */
if (pci_dev_present(pdev)) {
pr_info("PCI device hotplugged\n");
/* Perform device initialization */
initialize_pci_device(pdev);
} else {
pr_info("PCI device removed\n");
/* Perform cleanup */
pci_disable_device(pdev);
}
}
3. Advanced Features and Extensions:
- The Linux kernel provides various advanced features and extensions for PCI device management, including MSI-X support, SR-IOV, and PCIe hotplug. These features enable better performance, scalability, and flexibility in device management. Here's an example code snippet that demonstrates MSI-X initialization for a PCI device:
#include <linux/pci.h>
void initialize_msix(struct pci_dev *pdev)
{
/* Check if MSI-X is supported */
if (pci_msi_enabled()) {
pr_info("MSI-X supported, initializing...\n");
/* Perform MSI-X initialization */
pci_enable_msix(pdev, msix_entries, num_entries);
} else {
pr_info("MSI-X not supported\n");
}
}
Managing PCI devices and developing drivers for them in Linux requires a thorough understanding of device initialization, interrupt handling, memory management, power management, error handling, and advanced features. By following best practices and leveraging the rich set of APIs provided by the Linux kernel, developers can create robust and efficient drivers for a wide range of PCI devices.
#linuxdevicedrivers #ldd #linuxlovers
