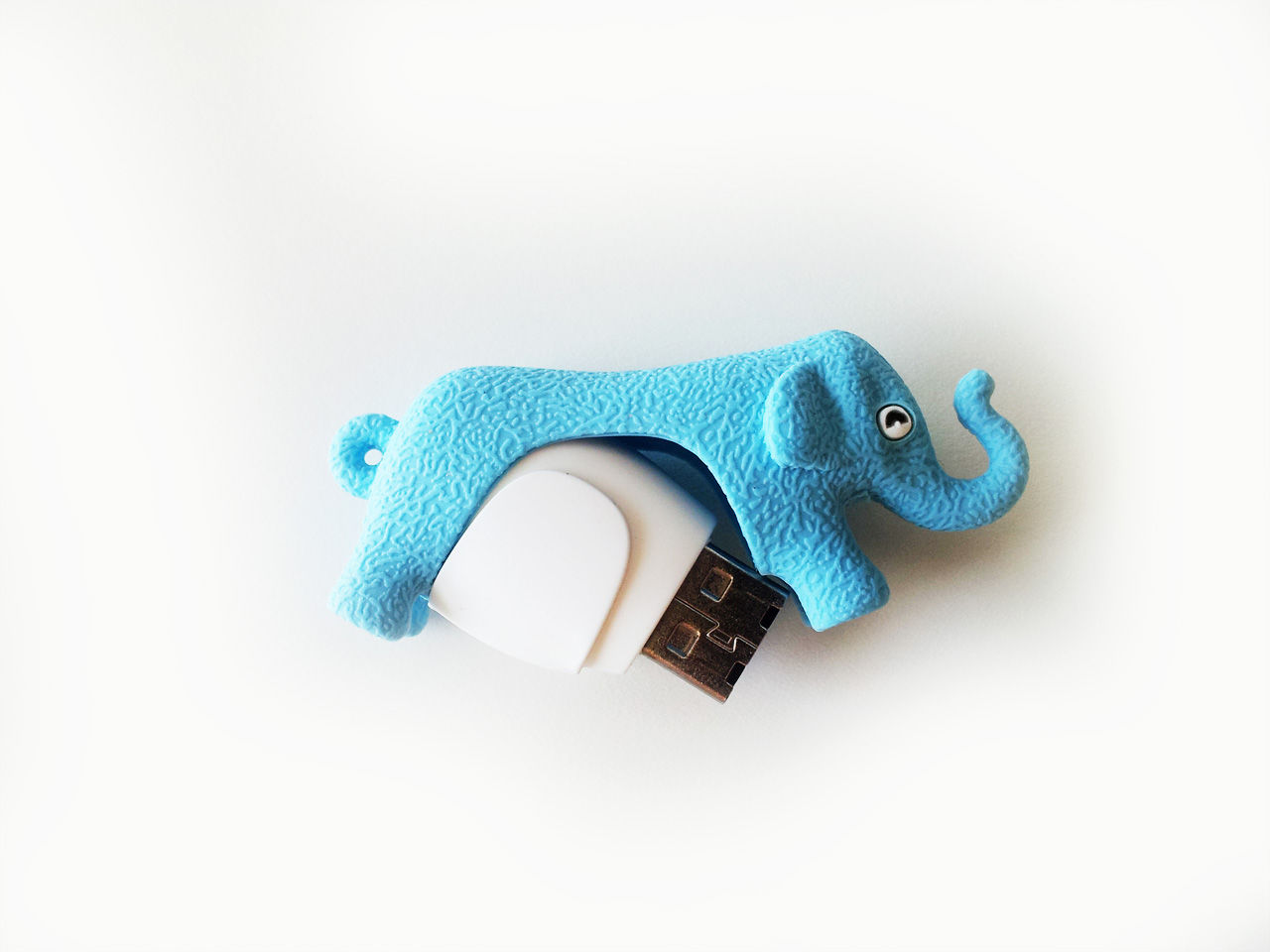
Continuing the Journey: Exploring Linux PCI in More Detail
- mkmints
- May 11, 2024
- 3 min read
Now that we've covered the basics of PCI in Linux, let's dive a little deeper into how it all works behind the scenes. In this continuation article, we'll explore some additional concepts and practical aspects of PCI management in the Linux operating system.
1. Device Enumeration Revisited:
In our previous article, we touched on the process of device enumeration, where the computer discovers all the PCI devices connected to it. Let's take a closer look at how this process unfolds and how the Linux kernel identifies and initializes each device it finds.
Example Function: `pci_scan_bus()`
/**
* pci_scan_bus - Scan a PCI bus, calling the callback for each device found
* @bus: the PCI bus to scan
* @fn: the callback function to call for each device
*
* Scan the specified PCI bus, calling the given callback function for each
* device found. The callback function should return zero to continue scanning
* or non-zero to stop.
*/
int pci_scan_bus(struct pci_bus bus, int (fn)(struct pci_dev , void ))
{
struct pci_dev *dev;
int error;
/* Iterate through each device on the bus */
list_for_each_entry(dev, &bus->devices, bus_list) {
/* Call the callback function for each device */
error = fn(dev, NULL);
if (error)
return error;
}
return 0;
}
2. Device Drivers in Action:
Device drivers play a crucial role in the PCI ecosystem, acting as intermediaries between the operating system and the hardware. In this section, we'll explore how device drivers are loaded, initialized, and utilized by the Linux kernel to facilitate communication with PCI devices.
Example Function: `pci_register_driver()`
/**
* pci_register_driver - register a driver with the PCI bus subsystem
* @driver: PCI driver structure
*
* Register a driver with the PCI bus subsystem. The driver's probe function
* will be called for each device that matches the driver's PCI device ID
* table.
*/
int pci_register_driver(struct pci_driver *driver)
{
/* Add the driver to the list of registered PCI drivers */
mutex_lock(&pci_driver_mutex);
list_add_tail(&driver->node, &pci_drivers);
mutex_unlock(&pci_driver_mutex);
return 0;
}
3. Resource Management Challenges:
Managing shared resources like memory and interrupts can be a complex task in PCI systems, especially when multiple devices are vying for access to the same resources. We'll delve into the strategies and mechanisms employed by the Linux kernel to efficiently allocate and manage resources among PCI devices.
Example Function: `pci_request_region()`
/**
* pci_request_region - request exclusive access to a PCI region
* @pdev: PCI device
* @region: PCI region to request
* @name: name of the requesting driver
*
* Request exclusive access to a PCI region for the given device. Returns
* zero on success or a negative error code on failure.
*/
int pci_request_region(struct pci_dev pdev, int region, const char name)
{
/* Check if the region is already claimed */
if (pci_resource_flags(pdev, region) & IORESOURCE_BUSY)
return -EBUSY;
/* Mark the region as busy */
pci_set_flag(pdev, region, IORESOURCE_BUSY);
return 0;
}
4. Handling Errors and Failures:
Even in the most well-designed systems, errors and failures can occur. In this section, we'll discuss how the Linux kernel handles errors and failures related to PCI devices, including techniques for error detection, recovery, and graceful degradation.
Example Function: `pci_enable_device()`
/**
* pci_enable_device - enable a PCI device and request resources
* @pdev: PCI device
*
* Enable a PCI device and request resources needed by the device. Returns
* zero on success or a negative error code on failure.
*/
int pci_enable_device(struct pci_dev *pdev)
{
/* Enable the PCI device */
if (pci_enable_device_flags(pdev, PCI_STD_RESOURCE_FLAGS))
return -ENODEV;
/* Request resources for the device */
if (pci_request_regions(pdev, DRV_NAME))
goto err_disable;
return 0;
err_disable:
pci_disable_device(pdev);
return -EBUSY;
}
As technology continues to evolve, so too does the field of PCI management in Linux. We'll explore some of the latest developments and emerging trends in PCI technology, such as PCIe Gen 5, NVMe over PCIe, and device virtualization, and discuss their implications for Linux PCI management.
By delving deeper into the intricacies of PCI management in Linux, we gain a better understanding of how our computer systems operate and how to optimize their performance and reliability. In this continuation article, we've scratched the surface of some advanced topics and provided a glimpse into the exciting future of Linux PCI management.
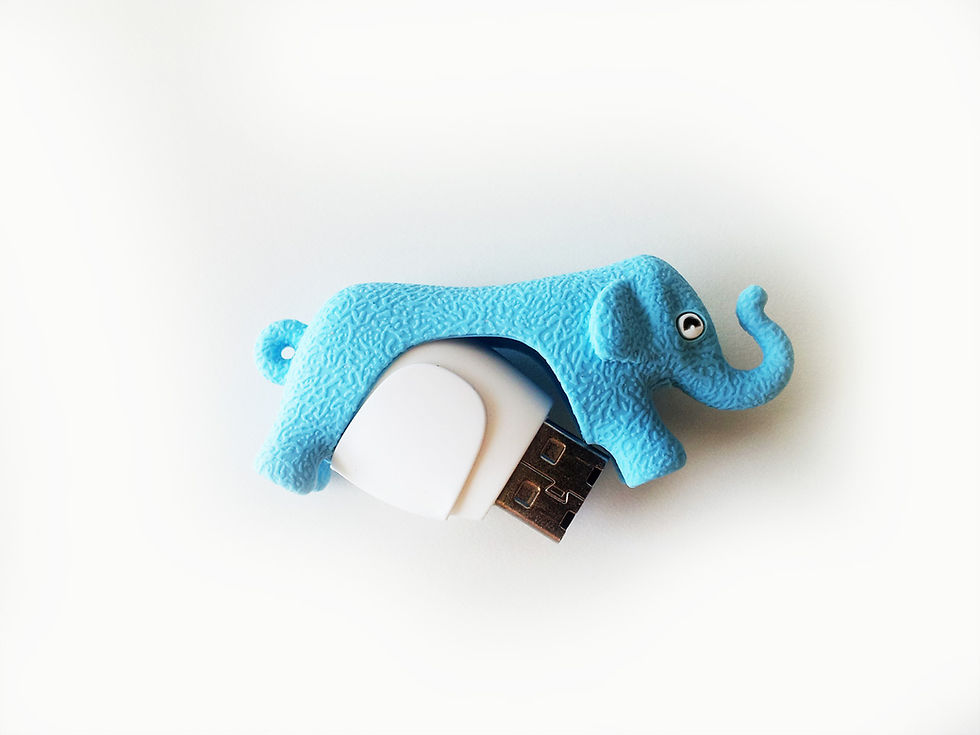