
Exploring Advanced Topics in Ethernet Driver Development
Apr 28, 2024
4 min read
0
69
In this article, we'll delve into advanced topics related to Ethernet driver development for the Linux kernel. Building upon the foundational knowledge covered in the previous article, we'll explore more complex concepts, provide detailed code examples, and explain their significance in the context of real-world networking scenarios.
1. Advanced Initialization and Configuration:
In the previous article, we initialized our Ethernet driver with basic functionality. In this section, we'll explore advanced initialization and configuration options, such as:
- Dynamic allocation of hardware resources
- Configuration of interrupt handling mechanisms
- Support for VLANs (Virtual Local Area Networks)
- Integration with advanced networking features like TCP/IP offloading
We'll see code examples demonstrating how to implement these features and discuss their impact on driver performance and functionality.
// Dynamic allocation of hardware resources
static int my_eth_probe(struct pci_dev *pdev, const struct pci_device_id *ent)
{
// Allocate memory regions, initialize hardware registers, etc.
return 0;
}
// Configuration of interrupt handling mechanisms
static irqreturn_t my_eth_interrupt(int irq, void *dev_id)
{
// Interrupt handling code
return IRQ_HANDLED;
}
// Support for VLANs (Virtual Local Area Networks)
static struct net_device *my_eth_alloc_netdev(void)
{
struct net_device *dev = alloc_netdev(sizeof(struct my_priv_data), "myeth%d", my_eth_setup);
if (dev) {
// Enable VLAN support
dev->features |= NETIF_F_HW_VLAN_TX | NETIF_F_HW_VLAN_RX;
}
return dev;
}
// Integration with advanced networking features
static void my_eth_setup(struct net_device *dev)
{
// Configure advanced features (e.g., offloading)
dev->features |= NETIF_F_HW_VLAN_FILTER | NETIF_F_RXCSUM;
}
2. Packet Transmission and Reception:
Efficient packet transmission and reception are essential for high-performance networking. In this section, we'll delve into advanced techniques for handling incoming and outgoing packets, including:
- Hardware acceleration for packet processing
- Integration with kernel networking subsystems, such as the network stack and socket interface
- Support for advanced packet filtering and classification mechanisms
- Optimization techniques for reducing latency and improving throughput
We'll illustrate these concepts with code snippets and discuss their implications for real-time and high-bandwidth networking applications.
// Hardware acceleration for packet processing
static netdev_tx_t my_eth_xmit(struct sk_buff *skb, struct net_device *dev)
{
// Transmit packet using hardware acceleration
return NETDEV_TX_OK;
}
// Integration with kernel networking subsystems
static int my_eth_rx(struct sk_buff *skb, struct net_device *dev, struct packet_type *pt, struct net_device *orig_dev)
{
// Process received packet using kernel networking subsystems
return 0;
}
// Support for advanced packet filtering and classification mechanisms
static void my_eth_filter(struct net_device *dev, struct sk_buff *skb)
{
// Apply advanced packet filtering rules
}
3. Power Management and Energy Efficiency:
Energy efficiency is becoming increasingly important in modern computing systems. In this section, we'll explore advanced techniques for power management and energy-efficient operation in Ethernet drivers, including:
- Dynamic power management (DPM) for reducing power consumption during idle periods
- Support for advanced sleep states (e.g., suspend-to-RAM) to minimize energy usage during periods of inactivity
- Integration with system-wide power management frameworks, such as ACPI (Advanced Configuration and Power Interface)
We'll provide code examples demonstrating how to implement these features and discuss their impact on overall system power consumption.
// Dynamic power management (DPM)
static int my_eth_suspend(struct device *dev)
{
// Put device into low-power state
return 0;
}
// Advanced sleep states (e.g., suspend-to-RAM)
static int my_eth_suspend_to_ram(struct device *dev)
{
// Suspend device to RAM
return 0;
}
// Integration with system-wide power management frameworks
static struct dev_pm_ops my_eth_pm_ops = {
.suspend = my_eth_suspend,
.suspend_to_ram = my_eth_suspend_to_ram,
};
4. Error Handling and Recovery:
Robust error handling and recovery mechanisms are crucial for maintaining network reliability and availability. In this section, we'll discuss advanced techniques for detecting, reporting, and recovering from errors in Ethernet drivers, including:
- Integration with kernel error reporting mechanisms, such as kernel logs and kernel crash dumps
- Support for advanced error recovery strategies, such as link re-negotiation and packet retransmission
- Implementation of fault-tolerant networking features, such as link aggregation and redundant link failover
We'll present code examples illustrating how to implement these features and discuss best practices for designing reliable and fault-tolerant Ethernet drivers.
// Integration with kernel error reporting mechanisms
static int my_eth_netdev_event(struct notifier_block *this, unsigned long event, void *ptr)
{
// Handle network device events (e.g., link status changes)
return NOTIFY_DONE;
}
// Advanced error recovery strategies
static void my_eth_error_recovery(struct net_device *dev)
{
// Implement error recovery logic (e.g., link re-negotiation)
}
// Fault-tolerant networking features
static void my_eth_link_aggregation(struct net_device *dev)
{
// Implement link aggregation for fault tolerance
}
5. Performance Optimization and Tuning:
Optimizing the performance of Ethernet drivers is essential for achieving maximum throughput and minimizing latency in networking applications. In this section, we'll explore advanced techniques for performance optimization and tuning, including:
- Fine-tuning of driver parameters and settings to optimize throughput, latency, and CPU utilization
- Integration with hardware offloading features for accelerating packet processing tasks
- Profiling and performance analysis techniques for identifying bottlenecks and optimizing critical code paths
- Support for multi-queue and multi-core processing to improve scalability and parallelism
We'll provide code examples demonstrating how to implement these techniques and discuss their impact on driver performance and scalability.
// Fine-tuning of driver parameters and settings
static void my_eth_optimize(struct net_device *dev)
{
// Adjust driver parameters for optimal performance
}
// Integration with hardware offloading features
static void my_eth_offload(struct net_device *dev)
{
// Enable hardware offloading features (e.g., checksum offload)
}
// Profiling and performance analysis techniques
static void my_eth_profile(void)
{
// Profile driver performance and identify bottlenecks
}
// Support for multi-queue and multi-core processing
static void my_eth_multi_queue(struct net_device *dev)
{
// Enable multi-queue support for improved scalability
}
In this article, we've explored advanced topics in Ethernet driver development for the Linux kernel. By understanding and implementing these concepts, developers can create high-performance, reliable, and energy-efficient Ethernet drivers that meet the demands of modern networking applications. With the provided code examples and explanations, readers can deepen their understanding of Ethernet driver development and apply these techniques to their own projects.
#linuxdevicedrivers #ldd #linuxlovers
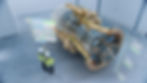