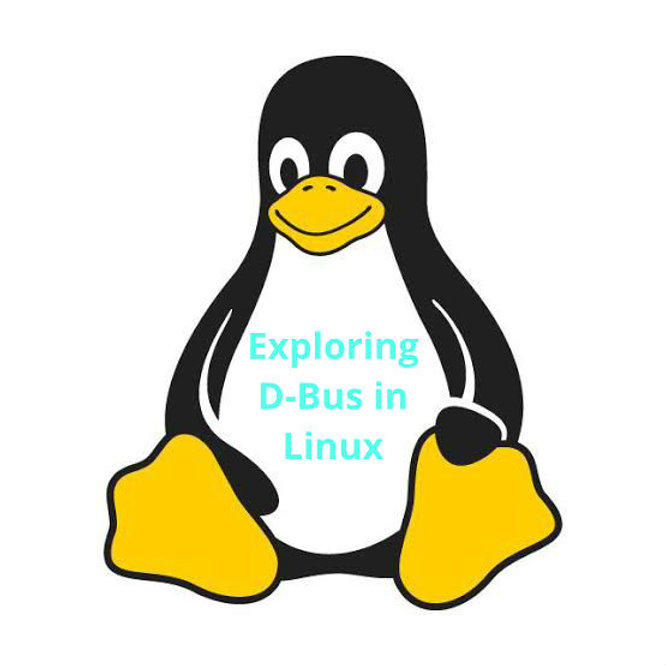
D-Bus is a message bus system that provides a simple and powerful inter-process communication (IPC) mechanism for applications running on Linux systems. In this article, we'll delve into the world of D-Bus, covering its fundamentals, architecture, usage, and practical examples to illustrate its usage in Linux programming.
1. Understanding D-Bus:
- D-Bus is a system for inter-process communication and coordination, enabling communication between multiple processes running on a Linux system.
- It allows applications to communicate with each other, regardless of the programming language they are written in, making it a versatile and widely-used IPC mechanism.
2. Architecture of D-Bus:
- D-Bus follows a client-server architecture, where the D-Bus daemon acts as a message bus between applications.
- Applications can register themselves with the D-Bus daemon as service providers, exposing their functionality through named objects called "interfaces".
- Other applications can then communicate with these services by sending messages to the D-Bus daemon, which routes them to the appropriate service.
3. Types of D-Bus:
- Session Bus: Used for communication between applications within the same user session.
- System Bus: Used for communication between system services and applications, accessible to all users on the system.
4. Creating and Using D-Bus Services:
- Service Registration: Use the D-Bus API to register a service with the D-Bus daemon, specifying the object path, interface, and methods exposed by the service.
- Message Passing: Use the D-Bus API to send and receive messages between applications, invoking methods on remote objects and handling signals.
5. Example: Creating a D-Bus Service in C
- Let's demonstrate how to create a simple D-Bus service in C:
#include <stdio.h>
#include <stdlib.h>
#include <dbus/dbus.h>
int main()
{
DBusError error;
dbus_error_init(&error);
// Connect to the D-Bus session bus
DBusConnection *connection = dbus_bus_get(DBUS_BUS_SESSION, &error);
if (dbus_error_is_set(&error)) {
fprintf(stderr, "Unable to connect to session bus: %s\n", error.message);
dbus_error_free(&error);
exit(EXIT_FAILURE);
}
// Register a service on the bus
const char *service_name = "com.example.SampleService";
const char *object_path = "/com/example/SampleObject";
dbus_bus_request_name(connection, service_name, 0, &error);
if (dbus_error_is_set(&error)) {
fprintf(stderr, "Failed to request service name: %s\n", error.message);
dbus_error_free(&error);
exit(EXIT_FAILURE);
}
// Main loop to handle incoming messages
while (dbus_connection_read_write_dispatch(connection, -1)) {}
return 0;
}
- D-Bus provides a flexible and powerful IPC mechanism for applications running on Linux systems.
- By understanding the fundamentals of D-Bus and its usage in Linux programming, developers can create sophisticated and interoperable applications.
- With practical examples, we've demonstrated how to create a D-Bus service in C, showcasing the simplicity and effectiveness of D-Bus in facilitating communication between processes.
#linuxdevicedrivers #ldd #linuxlovers
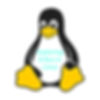