Exploring Filesystem Interface in Linux
- mkmints
- May 21, 2024
- 2 min read
The filesystem interface is a fundamental aspect of Linux, providing a structured approach for interacting with devices and resources through files. In this article, we'll delve into the intricacies of the filesystem interface, covering its architecture, common conventions, and practical examples to illustrate its usage.
1. Overview of Filesystem Interface:
- The filesystem interface in Linux treats devices and resources as files, facilitating uniform access using standard file I/O operations.
- Devices are represented as special files located within the `/dev` directory, while other resources may be accessible through various directories in the filesystem hierarchy.
2. Common Devices Represented as Files:
- Disk drives: `/dev/sda`, `/dev/sdb`
- Serial ports: `/dev/ttyS0`, `/dev/ttyS1`
- Audio devices: `/dev/snd/*`
- Input devices: `/dev/input/*`
3. File I/O Operations:
- `open()`: Opens a file for reading, writing, or both.
- `read()`: Reads data from an open file.
- `write()`: Writes data to an open file.
- `close()`: Closes an open file descriptor.
4. Example: Reading from a Serial Port (TTY):
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main()
{
int fd = open("/dev/ttyS0", O_RDONLY);
if (fd == -1) {
perror("Error opening serial port");
return 1;
}
char buffer[256];
ssize_t bytes_read = read(fd, buffer, sizeof(buffer));
if (bytes_read == -1) {
perror("Error reading from serial port");
close(fd);
return 1;
}
printf("Read %zd bytes: %s\n", bytes_read, buffer);
close(fd);
return 0;
}
5. Example: Writing to a File:
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main()
{
int fd = open("output.txt", O_WRONLY | O_CREAT | O_TRUNC, 0644);
if (fd == -1) {
perror("Error opening file");
return 1;
}
const char* message = "Hello, filesystem interface!";
ssize_t bytes_written = write(fd, message, strlen(message));
if (bytes_written == -1) {
perror("Error writing to file");
close(fd);
return 1;
}
printf("Wrote %zd bytes to file\n", bytes_written);
close(fd);
return 0;
}
- The filesystem interface in Linux provides a versatile means of accessing devices and resources through files.
- By leveraging standard file I/O operations, developers can interact with hardware peripherals and manage system resources efficiently.
- Understanding the filesystem interface is essential for building robust and scalable applications in the Linux ecosystem.
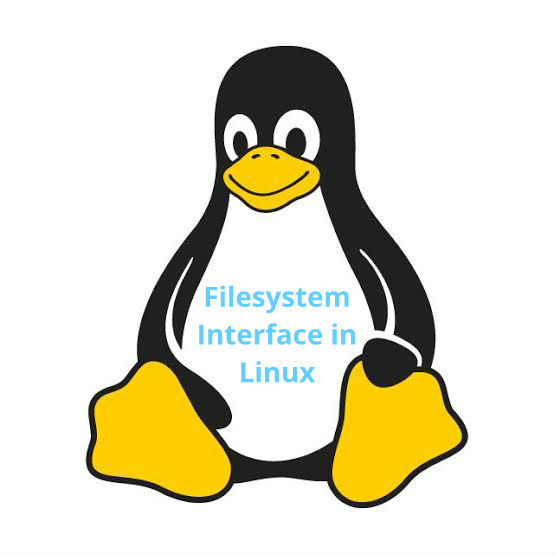