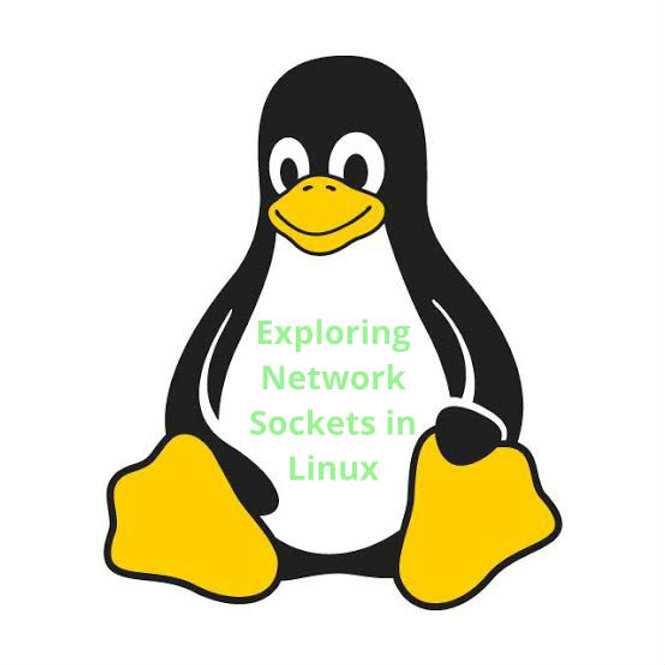
Network sockets are the cornerstone of network communication in Linux, providing a mechanism for processes to communicate with each other over the network. In this article, we'll delve into the world of network sockets, covering their fundamentals, types, creation, usage, and practical examples to illustrate their usage in Linux programming.
1. Understanding Network Sockets:
- A socket is an endpoint for communication between processes over a network.
- It is identified by an IP address and a port number, allowing processes to send and receive data over the network.
2. Types of Network Sockets:
- Stream Sockets (TCP): Provides reliable, connection-oriented communication between processes, ensuring data is delivered in the correct order and without loss.
- Datagram Sockets (UDP): Provides unreliable, connectionless communication between processes, suitable for applications where speed is more important than reliability.
3. Creating and Using Sockets:
- Socket Creation: Use the socket() system call to create a socket, specifying the address family (e.g., AF_INET for IPv4), socket type (e.g., SOCK_STREAM for TCP), and protocol (e.g., IPPROTO_TCP).
- Binding Sockets: Use the bind() system call to bind a socket to a specific IP address and port number.
- Listening for Connections (TCP): Use the listen() system call to put a socket into the listening state, allowing it to accept incoming connections.
- Accepting Connections (TCP): Use the accept() system call to accept incoming connections on a listening socket, creating a new socket for communication with the client.
- Sending and Receiving Data: Use the send() and recv() system calls (or their variants sendto() and recvfrom() for UDP) to send and receive data over the socket.
4. Example: TCP Server and Client
- Let's demonstrate how to create a simple TCP server and client in C:
Server:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <netinet/in.h>
int main()
{
int server_fd, new_socket;
struct sockaddr_in address;
int opt = 1;
int addrlen = sizeof(address);
// Create a TCP socket
if ((server_fd = socket(AF_INET, SOCK_STREAM, 0)) == 0) {
perror("socket failed");
exit(EXIT_FAILURE);
}
// Set socket options
if (setsockopt(server_fd, SOL_SOCKET, SO_REUSEADDR | SO_REUSEPORT, &opt, sizeof(opt))) {
perror("setsockopt");
exit(EXIT_FAILURE);
}
// Bind the socket to localhost and port 8080
address.sin_family = AF_INET;
address.sin_addr.s_addr = INADDR_ANY;
address.sin_port = htons(8080);
if (bind(server_fd, (struct sockaddr *)&address, sizeof(address)) < 0) {
perror("bind failed");
exit(EXIT_FAILURE);
}
// Listen for incoming connections
if (listen(server_fd, 3) < 0) {
perror("listen");
exit(EXIT_FAILURE);
}
// Accept incoming connection
if ((new_socket = accept(server_fd, (struct sockaddr *)&address, (socklen_t*)&addrlen)) < 0) {
perror("accept");
exit(EXIT_FAILURE);
}
// Send message to client
char *message = "Hello from server";
send(new_socket, message, strlen(message), 0);
printf("Message sent to client\n");
return 0;
}
Client:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <arpa/inet.h>
int main()
{
int sock = 0;
struct sockaddr_in serv_addr;
char buffer[1024] = {0};
// Create a TCP socket
if ((sock = socket(AF_INET, SOCK_STREAM, 0)) < 0) {
printf("\n Socket creation error \n");
return -1;
}
// Set server address and port
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = htons(8080);
// Convert IPv4 and IPv6 addresses from text to binary form
if(inet_pton(AF_INET, "127.0.0.1", &serv_addr.sin_addr) <= 0) {
printf("\nInvalid address/ Address not supported \n");
return -1;
}
// Connect to server
if (connect(sock, (struct sockaddr *)&serv_addr, sizeof(serv_addr)) < 0) {
printf("\nConnection Failed \n");
return -1;
}
// Receive message from server
read(sock, buffer, 1024);
printf("Message from server: %s\n", buffer);
return 0;
}
- Network sockets are a powerful mechanism for enabling communication between processes over a network in Linux.
- By understanding the fundamentals of sockets and their usage in Linux programming, developers can create robust and efficient networked applications.
- With practical examples, we've demonstrated how to create TCP server and client applications, showcasing the versatility and utility of network sockets in real-world scenarios.
#linuxdevicedrivers #ldd #linuxlovers
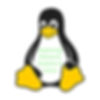