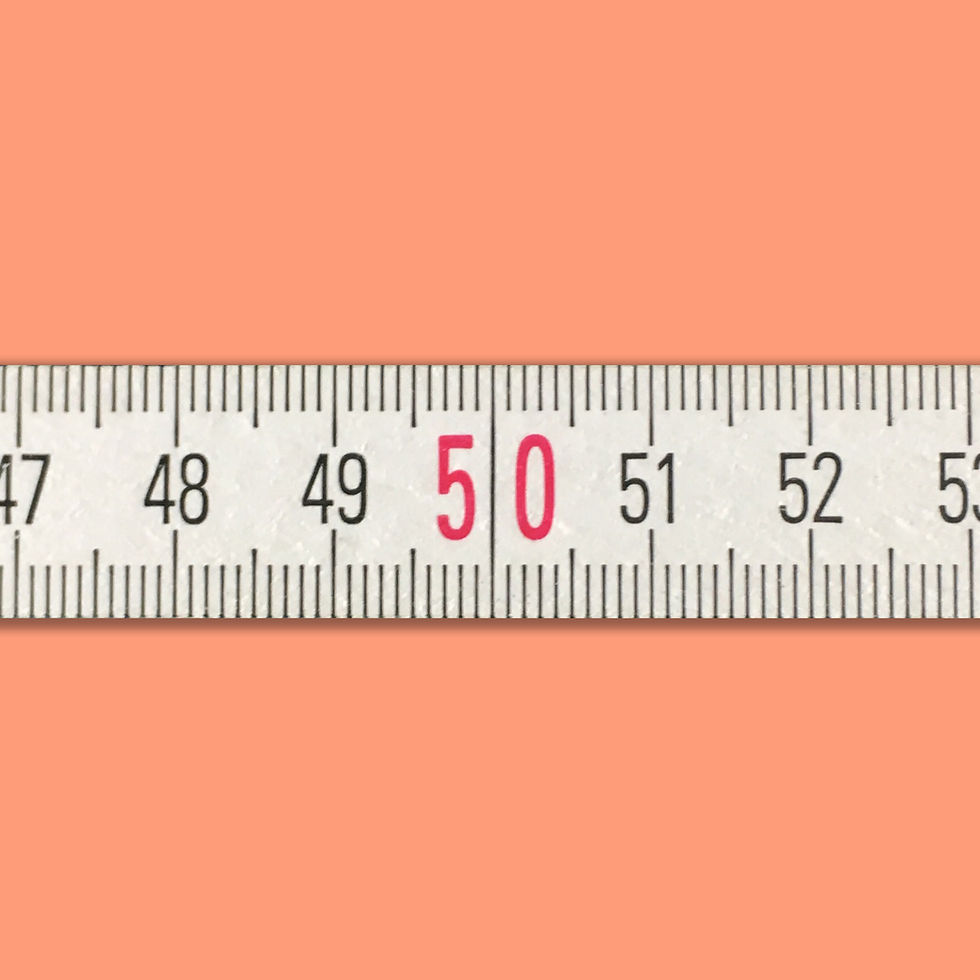
Exploring pthread APIs in Linux
- mkmints
- May 5, 2024
- 2 min read
Pthreads, or POSIX threads, are a popular threading model widely used in Linux for developing concurrent applications. In this article, we'll dive into the pthread APIs provided by the Linux kernel, covering thread creation, synchronization, and termination, along with examples to demonstrate their usage.
1. Thread Creation:
The pthread library provides functions for creating and managing threads. The `pthread_create()` function is used to create a new thread, specifying its entry point function and any arguments to be passed. Here's a basic example:
#include <pthread.h>
void* thread_function(void* arg) {
// Thread logic
return NULL;
}
int main() {
pthread_t thread;
pthread_create(&thread, NULL, thread_function, NULL);
pthread_join(thread, NULL); // Wait for thread to finish
return 0;
}
2. Thread Synchronization:
Pthreads offer various synchronization primitives for coordinating access to shared resources among threads. Mutexes (`pthread_mutex_t`), condition variables (`pthread_cond_t`), and semaphores (`sem_t`) are commonly used for this purpose. Here's an example of using a mutex to protect a shared resource:
#include <pthread.h>
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
int shared_resource = 0;
void* thread_function(void* arg) {
pthread_mutex_lock(&mutex);
shared_resource++;
pthread_mutex_unlock(&mutex);
return NULL;
}
int main() {
pthread_t thread1, thread2;
pthread_create(&thread1, NULL, thread_function, NULL);
pthread_create(&thread2, NULL, thread_function, NULL);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
return 0;
}
3. Thread Termination:
Threads can be terminated either voluntarily or involuntarily. Voluntary termination occurs when a thread reaches the end of its execution or calls `pthread_exit()`. Involuntary termination can be caused by signals or by another thread calling `pthread_cancel()`. Here's an example:
#include <pthread.h>
void* thread_function(void* arg) {
while (1) {
// Thread logic
pthread_testcancel(); // Check for cancellation
}
pthread_exit(NULL);
}
int main() {
pthread_t thread;
pthread_create(&thread, NULL, thread_function, NULL);
// Cancel thread after 5 seconds
sleep(5);
pthread_cancel(thread);
pthread_join(thread, NULL);
return 0;
}
4. Conclusion:
In this article, we've explored the pthread APIs provided by the Linux kernel for thread creation, synchronization, and termination. By leveraging these APIs, developers can create robust and efficient multithreaded applications in Linux, achieving concurrency and parallelism with ease. Experiment with these examples to deepen our understanding of pthreads and enhance your proficiency in Linux threading programming.
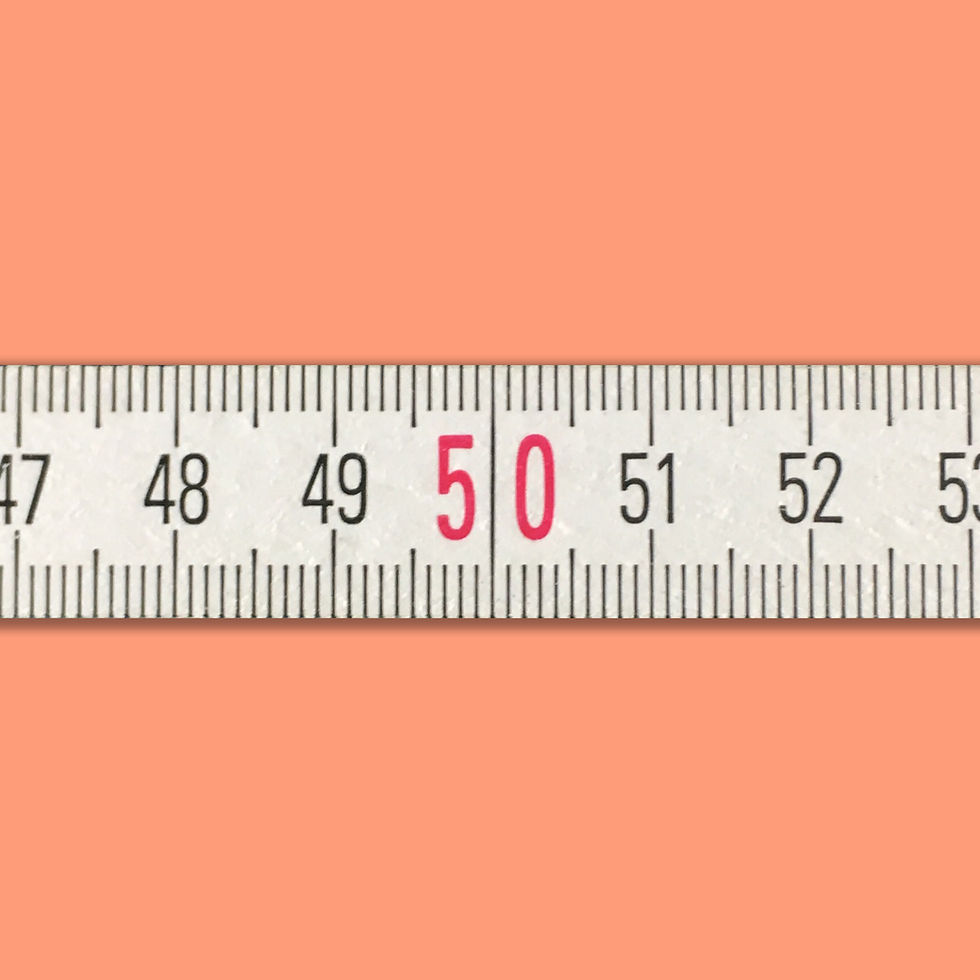