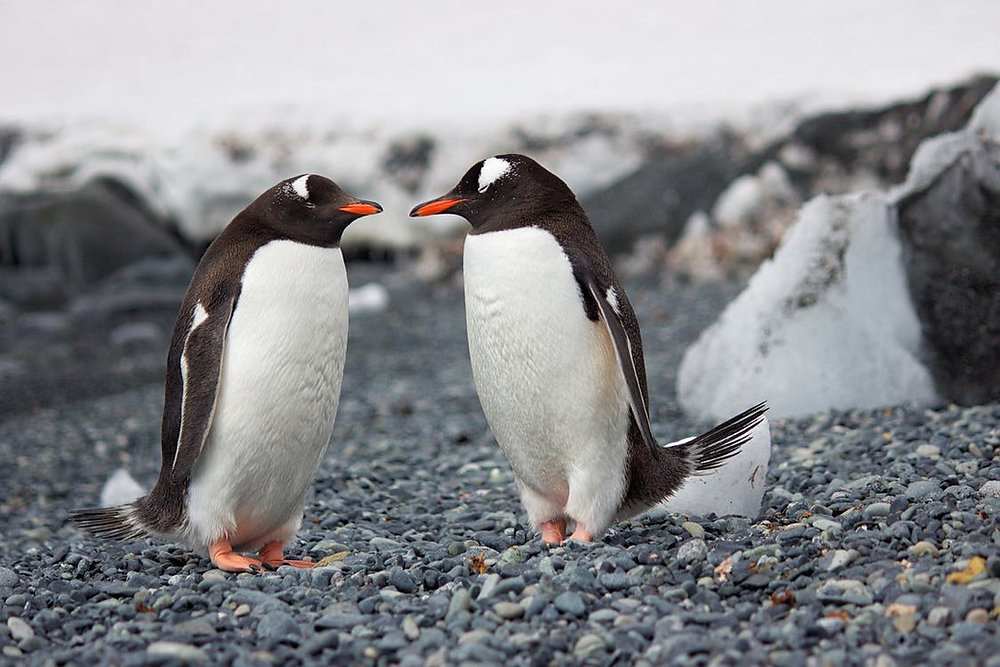
Exploring Thread Management in Linux Kernel
May 5, 2024
2 min read
2
119
Thread management is a crucial aspect of operating system design, and the Linux kernel provides robust mechanisms for creating, managing, and synchronizing threads. In this article, we'll delve into the intricacies of thread management in the Linux kernel, covering topics such as thread creation, scheduling, synchronization, and termination.
1. Thread Creation:
Threads in the Linux kernel are represented by the `task_struct` structure, which contains information about the thread's state, priority, and execution context. Threads can be created using system calls such as `clone()` or kernel functions like `kthread_create()`. These functions allow developers to specify various attributes of the new thread, including its entry point and stack size.
2. Scheduling:
The Linux kernel employs a multi-level scheduling algorithm to manage thread execution. Threads are scheduled based on their priority, with higher-priority threads preempting lower-priority ones. The scheduler maintains several queues of threads, such as the runqueue and waitqueue, to efficiently manage thread execution and synchronization.
3. Synchronization:
Thread synchronization is essential for ensuring data consistency and preventing race conditions in concurrent systems. The Linux kernel provides a variety of synchronization primitives, including mutexes, semaphores, spinlocks, and read/write locks. These primitives allow threads to coordinate access to shared resources and enforce mutual exclusion when necessary.
4. Termination:
Threads in the Linux kernel can be terminated either voluntarily or forcibly. Voluntary thread termination occurs when a thread reaches the end of its execution or calls the `exit()` system call. Forcible termination can be initiated by another thread using signals or by the kernel in response to exceptional conditions such as a kernel panic.
5. Code Example: Creating and Managing Threads:
Let's illustrate thread management in the Linux kernel with a simple code example:
#include <linux/kthread.h>
static int my_thread_func(void *data)
{
// Thread execution logic
return 0;
}
static struct task_struct *thread;
static int __init my_init(void)
{
// Create a new kernel thread
thread = kthread_run(my_thread_func, NULL, "my_thread");
if (IS_ERR(thread)) {
printk(KERN_ERR "Failed to create thread\n");
return PTR_ERR(thread);
}
return 0;
}
static void __exit my_exit(void)
{
// Terminate the kernel thread
if (thread)
kthread_stop(thread);
}
module_init(my_init);
module_exit(my_exit);
In this article, we've explored the fundamentals of thread management in the Linux kernel, covering thread creation, scheduling, synchronization, and termination. By understanding these concepts and utilizing the provided kernel APIs, developers can effectively manage threads in their Linux-based applications, ensuring efficient and reliable concurrent execution.
