
🔧 I2C Device Driver Tutorial: Part 6 - Advanced Features and Error Handling 🔧
Apr 11, 2024
1 min read
1
13
In the sixth part of our I2C device driver tutorial series, we'll explore advanced features and error handling techniques to enhance the reliability and functionality of our driver. Advanced features may include support for interrupts, device-specific commands, or power management, while error handling ensures robustness and resilience in the face of unexpected conditions. By mastering these techniques, we can develop high-quality I2C device drivers that meet the demands of real-world applications.
Implementing interrupt:
static irqreturn_t my_i2c_irq_handler(int irq, void *dev_id)
{
 // Handle interrupt event
 return IRQ_HANDLED;
}
static int my_i2c_setup_irq(struct i2c_client *client)
{
 int irq;
 // Setup interrupt handler
 irq = gpio_to_irq(client->irq_gpio);
 if (irq < 0) {
 dev_err(&client->dev, "Failed to map IRQ\n");
 return irq;
 }
 return request_irq(irq, my_i2c_irq_handler, IRQF_TRIGGER_FALLING, DEVICE_NAME, client);
}
static void my_i2c_cleanup_irq(struct i2c_client *client)
{
 int irq = gpio_to_irq(client->irq_gpio);
 free_irq(irq, client);
}
.
.
.
Explanation:
- The `my_i2c_irq_handler` function is called when an interrupt event occurs on the device. It handles the interrupt and returns IRQ_HANDLED.
- The `my_i2c_setup_irq` function maps the device's GPIO pin to an interrupt request (IRQ) and registers the interrupt handler.
- The `my_i2c_cleanup_irq` function releases the IRQ resources when the device is removed.
This example demonstrate how to integrate interrupt handling into our I2C device driver and provide error handling for IRQ setup and cleanup.
In the next part of the tutorial, we'll wrap up our I2C device driver development by discussing testing, debugging, and optimization strategies.
#linuxdevicedrivers #ldd #linuxlovers
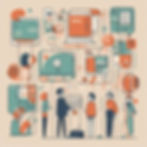