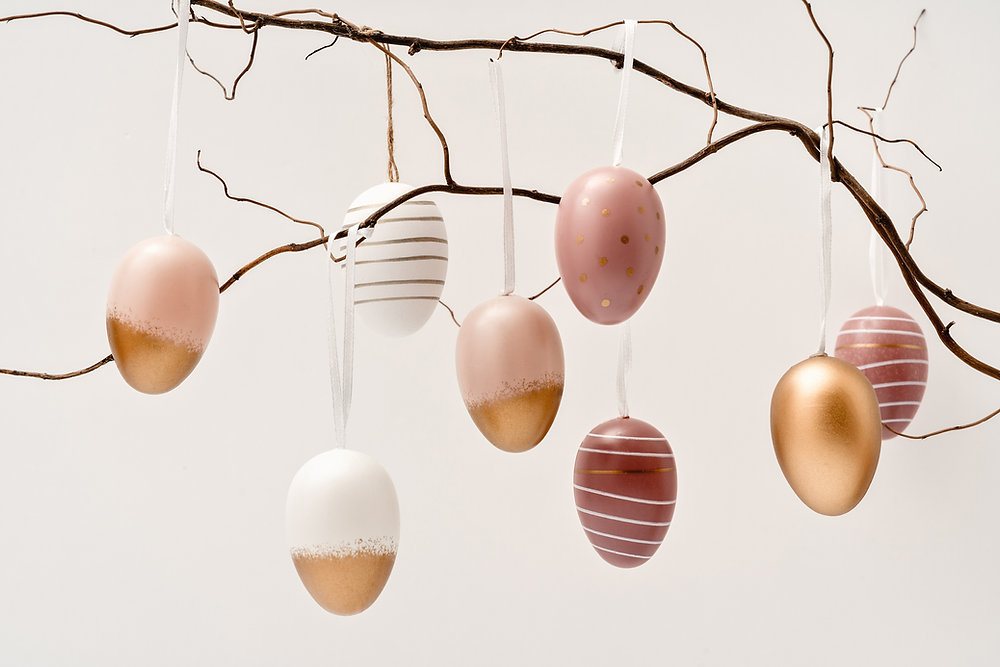
In Linux device driver development, the Input/Output Control (IOCTL) interface provides a powerful mechanism for communicating between user-space applications and kernel-space drivers. IOCTL commands allow user programs to send control requests and parameters to device drivers, enabling configuration, control, and interaction with hardware devices. In this article, we'll delve into the concept of IOCTL in Linux device drivers, explaining its usage, implementation, and providing examples to illustrate its practical application.
1. Understanding IOCTL:
IOCTL is a system call in Unix-like operating systems that allows user programs to perform input/output control operations on devices that do not fit into the standard read/write model. It provides a generic interface for sending commands, control codes, and data to device drivers, enabling various device-specific operations and configurations.
2. IOCTL Command Structure:
The IOCTL interface consists of three main components:
- Command: An integer value representing the specific operation or request to be performed by the device driver.
- Arguments: Optional parameters or data associated with the command, such as input/output buffers or control structures.
- Return Value: The result of the IOCTL operation, indicating success, failure, or additional information.
3. Implementing IOCTL in Device Drivers:
To support IOCTL commands, device drivers must implement an IOCTL handler function that interprets and processes IOCTL requests from user-space applications. This handler function typically resides within the device driver code and is invoked when an IOCTL command is received.
Example: IOCTL Handler Function
#include <linux/fs.h>
#include <linux/cdev.h>
#include <linux/ioctl.h>
long my_ioctl(struct file *filp, unsigned int cmd, unsigned long arg)
{
switch (cmd) {
case MY_IOCTL_COMMAND_1:
// Handle command 1
break;
case MY_IOCTL_COMMAND_2:
// Handle command 2
break;
default:
return -ENOTTY; // Invalid command
}
return 0; // Success
}
In this example:
- `my_ioctl` is the IOCTL handler function.
- `cmd` is the IOCTL command received from the user-space application.
- `arg` is an optional argument or parameter associated with the command.
4. Registering IOCTL Commands:
Before IOCTL commands can be used, they must be registered with the device driver and assigned unique command numbers. This is typically done using preprocessor macros or enums to define command codes within the driver code.
Example: IOCTL Command Definition
#define MY_IOCTL_MAGIC 'm'
#define MY_IOCTL_COMMAND_1 IO(MYIOCTL_MAGIC, 1)
#define MY_IOCTL_COMMAND_2 IO(MYIOCTL_MAGIC, 2)
In this example:
- `MY_IOCTL_MAGIC` is a unique identifier for the IOCTL commands.
- `MY_IOCTL_COMMAND_1` and `MY_IOCTL_COMMAND_2` are IOCTL command codes, each associated with a specific operation.
5. User-Space Interaction with IOCTL:
User-space applications can interact with device drivers using IOCTL commands through the `ioctl` system call. Applications pass the device file descriptor, IOCTL command code, and optional arguments to the `ioctl` function to initiate the operation.
Example: User-Space IOCTL Usage
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
int main()
{
int fd = open("/dev/mydevice", O_RDWR);
if (fd < 0) {
perror("Failed to open device");
return -1;
}
// Send IOCTL command 1
if (ioctl(fd, MY_IOCTL_COMMAND_1, 0) < 0) {
perror("IOCTL command 1 failed");
return -1;
}
close(fd);
return 0;
}
6. IOCTL Usage:
When implementing IOCTL in Linux device drivers, developers should consider the following best practices:
- Define clear and descriptive IOCTL command codes and parameters for ease of use and understanding.
- Validate user-provided arguments and parameters to prevent security vulnerabilities and ensure device stability.
- Document IOCTL usage and behavior in driver documentation to guide users and developers.
IOCTL provides a versatile interface for communication between user-space applications and kernel-space device drivers in Linux. By understanding the concepts and examples presented in this article, developers can leverage IOCTL effectively to implement device-specific operations, configurations, and interactions in their Linux-based systems.
#linuxdevicedrivers #ldd #linuxlovers
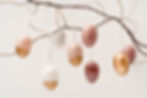