
Module parameters in Linux provide a mechanism for passing configuration options to kernel modules during module initialization. These parameters allow developers to customize the behavior of kernel modules without modifying the source code, enhancing flexibility and versatility. In this comprehensive guide, we'll explore the concept of Linux module parameters, their usage, and provide examples to illustrate their implementation.
1. Understanding Module Parameters:
Module parameters are variables defined within kernel modules that can be initialized with values provided by the user or system administrator during module loading. These parameters serve as tunable settings, allowing users to adjust module behavior or configure device-specific settings without recompiling the module.
2. Declaring Module Parameters:
Module parameters are typically declared using the `module_param` macro provided by the Linux kernel. This macro accepts several arguments, including the parameter name, data type, permissions, and optional callback functions for parameter validation and handling.
Example: Declaring Module Parameters
#include <linux/module.h>
#include <linux/moduleparam.h>
static int my_param = 0;
module_param(my_param, int, S_IRUGO);
MODULE_PARM_DESC(my_param, "Example module parameter");
In this example:
- `my_param` is the name of the module parameter.
- `int` specifies the data type of the parameter (in this case, an integer).
- `S_IRUGO` sets the permissions for the parameter to allow read-only access by user-space applications.
- `MODULE_PARM_DESC` provides a description of the parameter for documentation purposes.
3. Loading Module Parameters:
Module parameters can be specified when loading a kernel module using utilities like `insmod` or `modprobe`. Users can pass parameter values as key-value pairs separated by commas.
Example: Loading a Module with Parameters
insmod my_module.ko my_param=42
4. Accessing Module Parameters:
Once loaded, module parameters can be accessed and modified from user-space applications using utilities like `sysfs` or directly from kernel code using the `module_param_get` and `module_param_set` functions.
Example: Accessing Module Parameters from User-Space
cat /sys/module/my_module/parameters/my_param
echo 24 > /sys/module/my_module/parameters/my_param
5. Using Module Parameters in Kernel Code:
Kernel modules can access and use module parameters to customize their behavior or initialize internal variables based on user-provided values. Module parameters are typically accessed using global variables declared within the module.
Example: Using Module Parameters in Kernel Code
#include <linux/module.h>
extern int my_param;
static int __init my_module_init(void)
{
printk(KERN_INFO "My Module: Parameter Value = %d\n", my_param);
return 0;
}
6. Module Parameters:
When using module parameters in Linux kernel development, developers should consider the following best practices:
- Documenting module parameters with descriptive names and clear explanations of their purpose and usage.
- Validating parameter values within the module initialization function to ensure compatibility and prevent errors.
- Avoiding direct modification of module parameters by user-space applications unless necessary, to maintain system stability and security.
Linux module parameters provide a flexible and powerful mechanism for configuring kernel modules and customizing system behavior without modifying source code. By understanding the concepts and examples outlined in this guide, developers can leverage module parameters effectively to create modular and configurable kernel modules for a wide range of applications and use cases.
#linuxdevicedrivers #ldd #linuxlovers
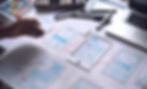