Linux Signals: Understanding and Harnessing the Power of Inter-Process Communication
May 30, 2024
3 min read
1
105
In the vast ecosystem of Linux, signals are akin to the silent messengers facilitating communication between processes. They serve as a fundamental mechanism for processes to convey critical information, respond to events, or gracefully terminate when necessary. Understanding Linux signals is not only essential for system developers but also for system administrators seeking to optimize system performance and reliability. In this comprehensive guide, we'll delve into the intricacies of Linux signals, explore their types, behaviors, and provide practical examples to demonstrate their usage.
Understanding Linux Signals:
At its core, a signal is a software interrupt that carries information about a specific event. It can be generated by the kernel, other processes, or by the process itself. Each signal is represented by a unique integer number, typically denoted by symbolic names prefixed with "SIG."
Types of Signals:
Linux signals can be broadly categorized into three main types:
1. Standard Signals (SIGINT, SIGTERM, SIGKILL, etc.): These signals have predefined meanings and default actions associated with them. For instance, SIGINT (signal interrupt) is typically triggered by pressing Ctrl+C, instructing a process to terminate gracefully.
2. Real-Time Signals (SIGRTMIN to SIGRTMAX): Unlike standard signals, real-time signals provide a more flexible mechanism for inter-process communication. They are not limited in number and can carry additional data along with the signal number.
3. Custom Signals: Developers have the flexibility to define their own signals beyond the standard and real-time signals. This allows for tailored communication between processes within an application or system
Signal Handling:
Signal handling refers to the process by which a process responds to incoming signals. There are three main actions that a process can take upon receiving a signal:
1. Default Action: Each signal has a default action associated with it, which is executed if the process does not specify a custom signal handler. For example, the default action for SIGTERM is to terminate the process.
2. Ignoring Signals: Processes can choose to ignore certain signals by explicitly specifying a signal handler that takes no action. This is often done for signals that are not relevant to the process or are being handled by a different mechanism.
3. Custom Signal Handling: Processes can define custom signal handlers using functions such as `signal()` or `sigaction()`. These handlers are executed when a specific signal is received, allowing processes to perform custom actions or handle the signal in a specific way.
Practical Examples:
Let's illustrate the usage of Linux signals with some practical examples:
1. Handling SIGINT:
#include <stdio.h>
#include <stdlib.h>
#include <signal.h>
void sigint_handler(int signum) {
printf("Received SIGINT signal. Exiting...\n");
exit(EXIT_SUCCESS);
}
int main() {
signal(SIGINT, sigint_handler);
printf("Press Ctrl+C to terminate.\n");
while(1) {
// Do some work
}
return 0;
}
2. Sending Custom Signal:
#include <stdio.h>
#include <signal.h>
void sigusr1_handler(int signum) {
printf("Received SIGUSR1 signal.\n");
}
int main() {
struct sigaction sa;
sa.sa_handler = sigusr1_handler;
sigemptyset(&sa.sa_mask);
sa.sa_flags = 0;
sigaction(SIGUSR1, &sa, NULL);
printf("Sending SIGUSR1 signal...\n");
kill(getpid(), SIGUSR1);
return 0;
}
Linux signals form the backbone of inter-process communication and event handling in the Linux environment. By understanding the different types of signals, their behaviors, and signal handling mechanisms, developers and system administrators can harness the power of signals to build robust and responsive systems. Whether gracefully terminating processes, implementing custom inter-process communication, or responding to specific events, signals play a vital role in the seamless operation of Linux-based systems.
#linuxdevicedrivers #ldd #linuxlovers
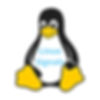