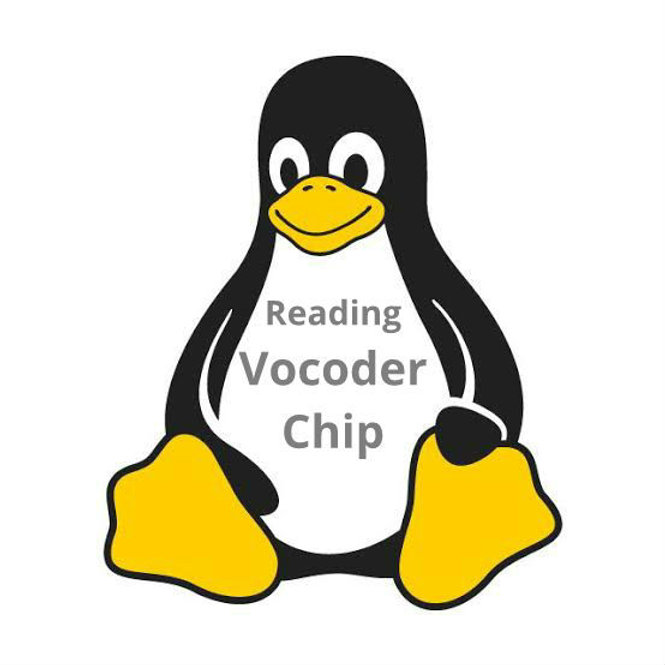
Reading from UART Vocoder Chip from Linux
Jun 15, 2024
2 min read
1
23
UART Vocoder chips are integral components in voice processing systems, facilitating voice encoding and decoding tasks. This article offers a comprehensive guide on how to read data from a UART Vocoder chip using a Linux-based system. We'll cover the necessary steps, including device detection, UART configuration, data reception, and provide example code snippets for practical implementation.
1. Device Detection and Identification:
Before reading data from the UART Vocoder chip, it's essential to detect and identify the connected device. Linux provides support for UART devices through serial drivers, which automatically detect connected UART devices. Tools like "dmesg" or examining the "/dev" directory can help identify the UART device corresponding to the Vocoder chip.
2. UART Configuration:
Once the UART Vocoder chip is identified, configure the UART interface settings such as baud rate, data bits, stop bits, and parity. Use tools like stty or IOCTL calls in C/C++ to set UART parameters. Ensure that the UART settings match the specifications of the Vocoder chip for proper communication.
3. Data Reception:
After configuring the UART interface, read data from the UART Vocoder chip. Data reception involves continuously polling or waiting for incoming data on the UART interface. Upon receiving data, process it accordingly for further analysis or storage.
4. Example Code Snippets:
Below are example code snippets demonstrating how to configure the UART interface and read data from a UART Vocoder chip in Linux:
Example 1: UART Configuration in C/C++
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int main()
{
int uart_fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (uart_fd < 0) {
perror("Error opening UART device");
return -1;
}
struct termios options;
tcgetattr(uart_fd, &options);
// Set baud rate to 9600
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
// Set data bits to 8, no parity, 1 stop bit
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(uart_fd, TCSANOW, &options);
close(uart_fd);
return 0;
}
Example 2: Reading Data from UART in C/C++
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#define BUFFER_SIZE 1024
int main()
{
int uart_fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (uart_fd < 0) {
perror("Error opening UART device");
return -1;
}
char data_buffer[BUFFER_SIZE];
// Read data from UART
ssize_t bytes_read = read(uart_fd, data_buffer, BUFFER_SIZE);
if (bytes_read < 0) {
perror("Error reading from UART device");
close(uart_fd);
return -1;
}
// Process received data
// ...
close(uart_fd);
return 0;
}
Reading data from a UART Vocoder chip in Linux involves device detection, UART configuration, and data reception. By following the steps outlined in this guide and using the example code snippets provided, developers can effectively read data from UART Vocoder chips in Linux-based systems, enabling various voice processing applications and functionalities.
#linuxdevicedrivers #ldd #linuxlovers
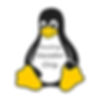