
The Mouse That Roared: A Comedic Tale of Linux Kernel Handling
Apr 20, 2024
3 min read
0
99
Once upon a Linux kernel, in a kingdom of ones and zeros, there lived a curious creature known as the mouse. But this wasn't your ordinary mouse, scurrying around in search of cheese. No, this mouse was a digital marvel, with buttons and sensors, ready to navigate the vast digital landscape of computer screens.
The Mouse's Adventure Begins
Our story begins with the humble mouse device, innocently plugged into a computer's USB port. Little did it know, it was about to embark on a wild journey through the depths of the Linux kernel.
// Kernel detects the mouse device
void detect_mouse_device() {
printk(KERN_INFO "Hello, little mouse! I've detected your presence.\n");
}
// Kernel loads the appropriate driver module
void load_mouse_driver() {
printk(KERN_INFO "Loading mouse driver module...\n");
// Code to load the driver module goes here
}
// Kernel initializes mouse driver functions
void initialize_mouse_driver() {
printk(KERN_INFO "Initializing mouse driver functions...\n");
// Code to initialize driver functions goes here
}
// Driver function to handle mouse movement
void handle_mouse_movement(int x, int y) {
printk(KERN_INFO "Mouse moved to coordinates: (%d, %d)\n", x, y);
}
// Driver function to handle mouse button clicks
void handle_mouse_click(int button) {
printk(KERN_INFO "Mouse clicked button %d\n", button);
}
// Driver function to handle mouse wheel scrolls
void handle_mouse_scroll(int direction) {
printk(KERN_INFO "Mouse scrolled %s\n", direction > 0 ? "up" : "down");
}
Relationship of Functions:
- When the kernel detects the mouse device, it triggers the `detect_mouse_device()` function to acknowledge its presence.
- Subsequently, the kernel initiates the loading of the appropriate mouse driver module using the `load_mouse_driver()` function.
- After loading the driver, the kernel initializes the driver's functions, including handling mouse movement, clicks, and scrolls, through the `initialize_mouse_driver()` function.
- Once initialized, the driver functions are ready to be invoked whenever the mouse interacts with the system.
Driver Source File (mouse_driver.c):
#include <linux/module.h>
#include <linux/kernel.h>
// Function prototypes
void detect_mouse_device();
void load_mouse_driver();
void initialize_mouse_driver();
void handle_mouse_movement(int x, int y);
void handle_mouse_click(int button);
void handle_mouse_scroll(int direction);
// Module initialization
static int __init mouse_driver_init(void) {
detect_mouse_device();
load_mouse_driver();
initialize_mouse_driver();
return 0;
}
// Module cleanup
static void __exit mouse_driver_exit(void) {
printk(KERN_INFO "Unloading mouse driver module...\n");
// Code to unload the driver module goes here
}
module_init(mouse_driver_init);
module_exit(mouse_driver_exit);
MODULE_LICENSE("GPL");
MODULE_AUTHOR("LinuxLovers-mkmints");
MODULE_DESCRIPTION("Linux kernel mouse driver");
- The Kernel's Inner Workings: Behind the scenes, the kernel employs various data structures and algorithms to manage the mouse device and its interactions with the system. From interrupt handling to event processing, each aspect of mouse handling involves intricate kernel mechanisms working in harmony.
- Driver Development Challenges: Writing a mouse driver isn't all fun and games. Developers face challenges such as hardware compatibility, performance optimization, and ensuring seamless integration with the kernel. But with dedication and creativity, they overcome these hurdles to deliver reliable and efficient drivers.
- The Mouse's Legacy: As technology evolves, so too does the humble mouse. From traditional wired mice to wireless and ergonomic designs, the mouse continues to adapt to the changing needs of users. And through it all, the Linux kernel stands ready to handle whatever the mouse throws its way.
In the whimsical world of Linux, even the simplest of devices can become the protagonists of fantastical tales. So, the next time you click your mouse or scroll your wheel, remember the magical journey it takes through the enchanting realm of the Linux kernel.
#linuxdevicedrivers #ldd #linuxlovers
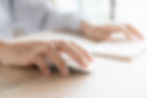