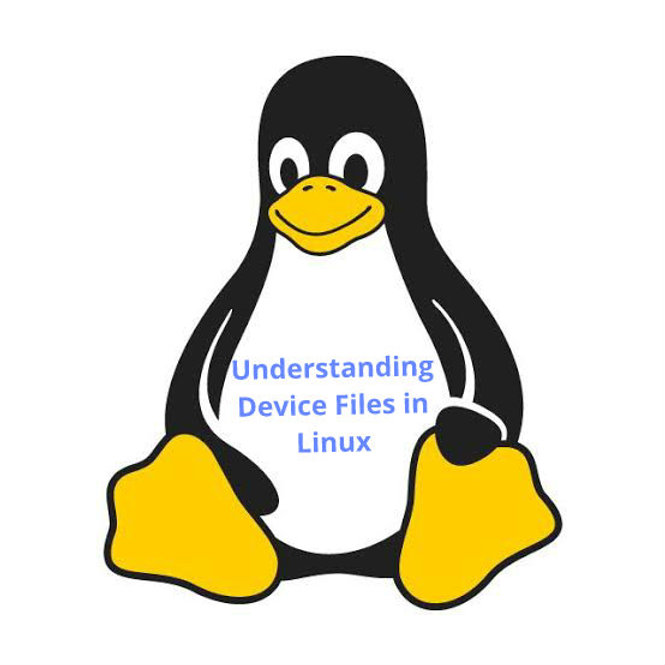
Device files play a crucial role in Linux systems, providing a standardized interface for accessing hardware devices and resources. In this article, we'll explore the concept of device files, their significance, and practical examples to demonstrate their usage.
1. What are Device Files?
- Device files, also known as special files, represent hardware devices and resources within the Linux filesystem.
- They serve as a bridge between user-space applications and kernel drivers, facilitating communication and control over hardware peripherals.
2. Types of Device Files:
- Character Devices: Represent devices that stream data character by character, such as serial ports and input devices.
- Block Devices: Represent devices that store and retrieve data in fixed-size blocks, such as hard drives and flash memory.
3. Device File Naming Convention:
- Device files are typically located in the `/dev` directory, organized based on device type and number.
- Character devices are named `cXn`, where `X` represents the major device number and `n` represents the minor device number.
- Block devices are named `bXn`, following the same convention.
4. Example: Accessing a Serial Port (Character Device)
- Let's demonstrate how to read data from a serial port (`/dev/ttyS0`) using a C program:
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main()
{
int fd = open("/dev/ttyS0", O_RDONLY);
if (fd == -1) {
perror("Error opening serial port");
return 1;
}
char buffer[256];
ssize_t bytes_read = read(fd, buffer, sizeof(buffer));
if (bytes_read == -1) {
perror("Error reading from serial port");
close(fd);
return 1;
}
printf("Read %zd bytes: %s\n", bytes_read, buffer);
close(fd);
return 0;
}
5. Example: Accessing a Disk Drive (Block Device)
- Let's demonstrate how to read data from a disk drive (`/dev/sda`) using a C program:
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main()
{
int fd = open("/dev/sda", O_RDONLY);
if (fd == -1) {
perror("Error opening disk drive");
return 1;
}
// Read data from disk drive and process as needed
close(fd);
return 0;
}
- Device files serve as essential components in Linux systems, providing a standardized interface for accessing hardware devices and resources.
- By understanding device files and their naming conventions, developers can interact with hardware peripherals efficiently and effectively.
- With practical examples, we've demonstrated how to access both character and block devices using C programs, showcasing the versatility of device files in Linux.
#linuxdevicedrivers #ldd #linuxlovers
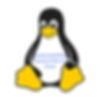