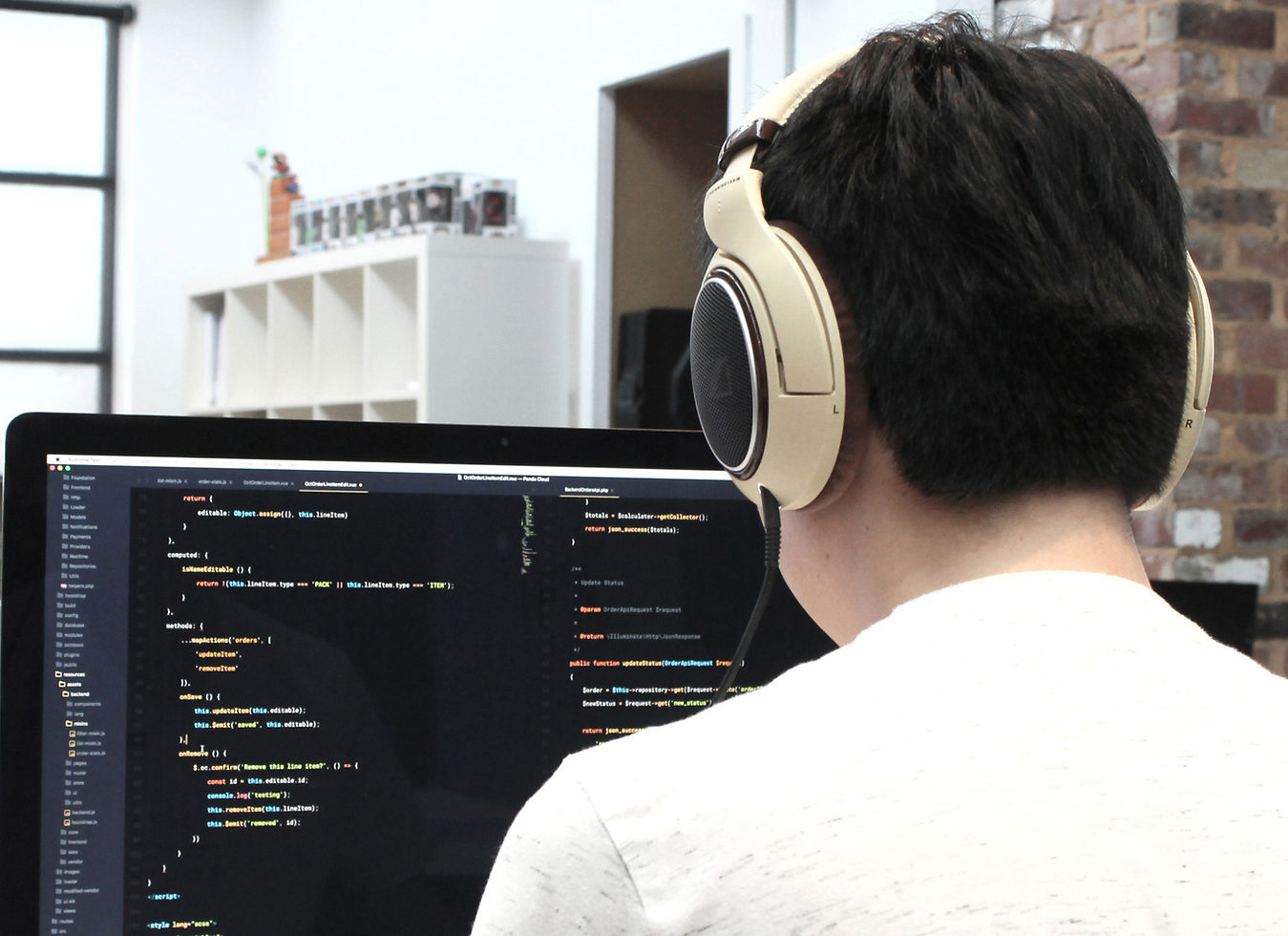
Understanding Program Execution in Linux
- mkmints
- May 5, 2024
- 2 min read
In the Linux operating system, program execution involves several stages, from loading the program into memory to running it on the CPU. In this article, we'll explore the intricacies of program execution in Linux, covering topics such as program loading, process creation, execution modes, and dynamic linking, along with detailed examples to illustrate each stage.
1. Program Loading:
When a program is executed in Linux, the kernel loads it into memory from the disk. This involves several steps, including locating the program binary, allocating memory space, and setting up the program's execution environment. The `execve()` system call is commonly used to load and execute programs in Linux.
2. Process Creation:
Once the program is loaded into memory, the kernel creates a new process to execute it. Process creation involves duplicating the current process's memory space and assigning it to the new process. The `fork()` system call is often used to create new processes in Linux, followed by the `execve()` system call to replace the new process's memory space with the program's image.
3. Execution Modes:
Linux supports two main execution modes for running programs: user mode and kernel mode. In user mode, programs have limited access to system resources and must use system calls to request services from the kernel. In kernel mode, programs have unrestricted access to system resources and can execute privileged instructions. The transition between user mode and kernel mode is controlled by the CPU's hardware mechanisms.
4. Dynamic Linking:
Many programs in Linux make use of dynamic linking, where library functions are linked to the program at runtime rather than at compile time. This allows programs to share common libraries and reduces executable size. Dynamic linking is facilitated by the dynamic linker (`ld.so`) and the dynamic linker loader (`ld-linux.so`), which resolve library dependencies and load shared libraries into the program's address space.
5. Example:
Here's a simple C program demonstrating program execution in Linux:
#include <stdio.h>
int main() {
printf("Hello, LinuxLovers-mkmints!\n");
return 0;
}
To compile and execute the program:
gcc -o hello hello.c
./hello
In this article, we've explored the various stages of program execution in Linux, including program loading, process creation, execution modes, and dynamic linking. By understanding these concepts and mechanisms, developers can gain insights into how programs are executed in Linux and optimize their code for performance and efficiency. Experiment with the provided examples to deepen your understanding and proficiency in Linux programming.
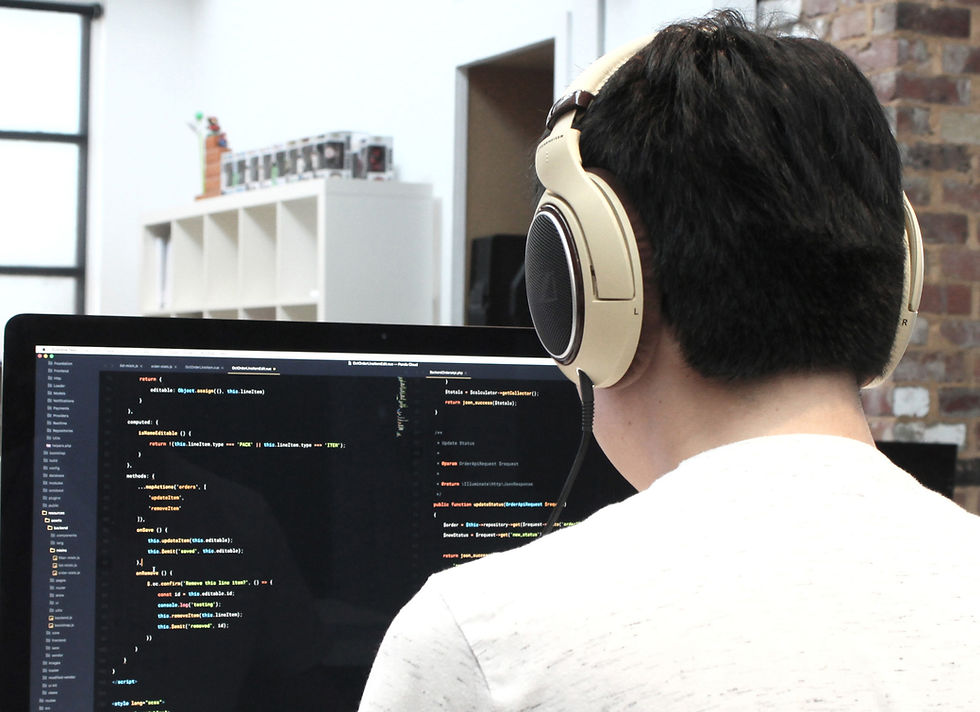