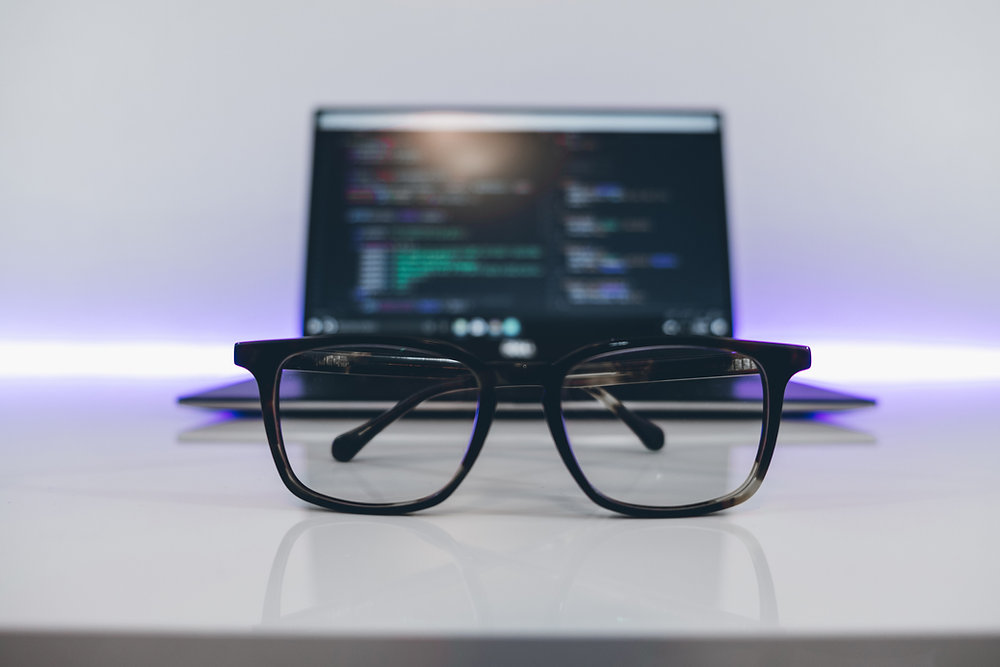
Writing Your First Ethernet Driver: A Step-by-Step Guide
Apr 28, 2024
3 min read
2
546
In this article, we'll embark on a journey to write our first Ethernet driver for the Linux kernel. We'll start from scratch, exploring the essential components of an Ethernet driver and gradually building up our driver's functionality. By the end of this guide, you'll have a solid understanding of how Ethernet drivers are implemented in the Linux kernel and the steps involved in writing your own driver.
Understanding the Basics:
Before diving into driver development, let's take a moment to understand the basics of Ethernet networking and how it relates to kernel drivers. Ethernet is a widely used networking technology that allows devices to communicate over a local area network (LAN). In the Linux kernel, Ethernet drivers are responsible for managing network interfaces and facilitating the transmission and reception of data packets.
Setting Up Your Development Environment:
To begin writing our Ethernet driver, we'll need a development environment with the necessary tools and libraries installed. We'll use a Linux-based system with the kernel source code available for reference. You can download the kernel source code from the official Linux kernel repository or use a pre-built kernel package provided by your distribution.
Creating the Driver Skeleton:
Our first step is to create the basic structure of our Ethernet driver. We'll start by defining the necessary includes and declaring the initialization and exit functions for our driver. Here's a simplified version of our driver skeleton:
#include <linux/module.h>
#include <linux/netdevice.h>
static int my_eth_init(struct net_device *dev)
{
// Initialization code goes here
printk(KERN_INFO "Initializing My Ethernet driver\n");
// Assign MAC address
ether_setup(dev);
dev->netdev_ops = &my_netdev_ops;
dev->flags |= IFF_PROMISC;
return 0;
}
static void my_eth_exit(struct net_device *dev)
{
// Cleanup code goes here
printk(KERN_INFO "Exiting My Ethernet driver\n");
}
static struct net_device_ops my_netdev_ops = {
.ndo_init = my_eth_init,
.ndo_uninit = my_eth_exit,
// Add more operations here
};
static int __init my_eth_init_module(void)
{
struct net_device *dev = alloc_netdev(0, "myeth%d", NET_NAME_UNKNOWN, ether_setup);
if (!dev)
return -ENOMEM;
register_netdev(dev);
printk(KERN_INFO "My Ethernet driver registered\n");
return 0;
}
static void __exit my_eth_exit_module(void)
{
// Cleanup and unregister code goes here
struct net_device *dev = dev_get_by_name(&init_net, "myeth0");
if (!dev) {
printk(KERN_ERR "Ethernet device not found\n");
return;
}
unregister_netdev(dev);
free_netdev(dev);
printk(KERN_INFO "My Ethernet driver unregistered\n");
}
module_init(my_eth_init_module);
module_exit(my_eth_exit_module);
MODULE_LICENSE("GPL");
MODULE_AUTHOR("LinuxLovers-mkmints");
MODULE_DESCRIPTION("Sample Ethernet driver for Linux");
In the above code:
- We've added more initialization code in the `my_eth_init` function, including setting up the MAC address, assigning network device operations, and enabling promiscuous mode.
- In the `my_eth_exit` function, we've added cleanup code to print a message when the driver exits.
- We've defined additional network device operations in the `my_netdev_ops` structure. You can add more operations as needed for your driver.
- In the `my_eth_init_module` function, we've allocated and registered the network device, and printed a message to indicate successful registration.
- In the `my_eth_exit_module` function, we've added code to unregister and free the network device when the driver is unloaded, and printed a corresponding message.
With these additions, our Ethernet driver now has more functionality and robustness.
Compiling and Loading the Driver:
Once we've written our driver code, we'll compile it into a loadable kernel module (LKM) using the appropriate build tools. We'll then load the module into the kernel using the `insmod` command and verify that it's successfully registered with the kernel.
Testing the Driver:
With our driver loaded into the kernel, we'll test its functionality by interacting with it through user-space utilities or by simulating network traffic. We'll verify that the driver initializes correctly, handles network packets, and cleans up resources when unloaded from the kernel.
In this article, we've covered the basics of writing an Ethernet driver for the Linux kernel. We've explored the essential components of a driver, created a driver skeleton, and discussed the key functions involved in driver initialization and cleanup. Armed with this knowledge, you're ready to dive deeper into Linux kernel driver development and explore more advanced topics. Happy coding!
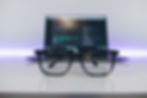